C++ Exercises: Replace all the words "dog" with "cat"
Replace "dog" with "cat"
Write a C++ program that replaces all the words "dog" with "cat".
Sample Text: The quick brown fox jumps over the lazy dog. You can assume that the number of characters in a text is less than or equal to 1000
.
Visual Presentation:
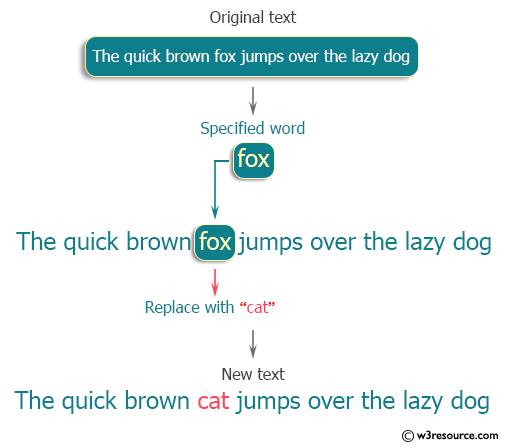
Sample Solution:
C++ Code:
#include <iostream>
using namespace std;
int main()
{
string str;
getline(cin, str); // Input text from the user
cout << "Original text: " << str; // Display original text
// Iterate through the string
for (int j = 0; j < (int)str.size(); j++) {
string key = str.substr(j, 3), repl; // Extract a substring of length 3
if (key == "fox") { // Check if the extracted substring matches "fox"
repl = "cat"; // If matched, replace with "cat"
for (int k = 0; k < 3; k++) {
str[j + k] = repl[k]; // Replace the matched substring in the original text
}
}
}
cout << "\nNew text: " << str << endl; // Display the modified text
return 0;
}
Sample Output:
Original text: The quick brown fox jumps over the lazy dog New text: The quick brown cat jumps over the lazy dog
Flowchart:
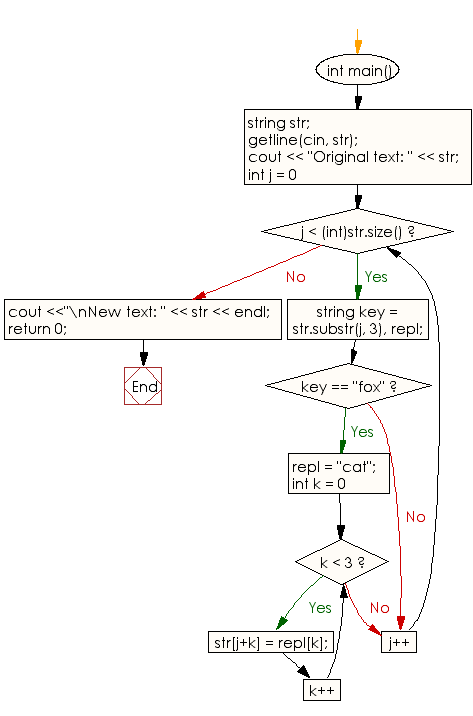
C++ Code Editor:
Previous: Write a C++ program that accepts n different numbers (0 to 100) and s which is equal to the sum of the n different numbers.
Next: Write a C++ program which reads a list of pairs of a word and a page number, and prints the word and a list of the corresponding page numbers.
What is the difficulty level of this exercise?
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics