C++ Exercises: Accepts n different numbers and s which is equal to the sum of the n different numbers
Combinations for Given Sum
Write a C++ program that accepts n different numbers (0 to 100) as well as an integer s which is equal to the sum of the n different numbers.
Your job is to find the number of combinations of n numbers and the same number cannot be used for one combination
Example:
Here n = 3 and s = 6:
1 + 2 + 3 = 6
0 + 1 + 5 = 6
0 + 2 + 4 = 6
Output: Number of combination: 3
Visual Presentation:
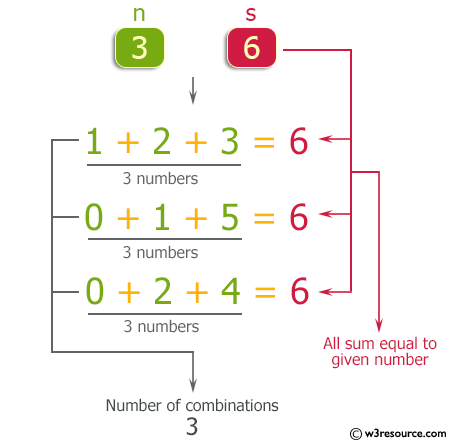
Sample Solution:
C++ Code :
#include <iostream>
// Macros for defining range loops
#define range(i,a,b) for(int (i)=(a);(i)<(b);(i)++)
#define rep(i,n) range(i,0,n)
using namespace std;
int n, s;
long long int dp[10][1010]; // Declaration of 2D array to store combinations
int main(void) {
dp[0][0] = 1LL; // Initialize the first element of the array to 1
// Loop for calculating combinations
rep(i, 101) {
for (int j = 8; j >= 0; j--) {
rep(k, 1010) {
if (k + i <= 1010) {
dp[j + 1][k + i] += dp[j][k]; // Calculate combinations and store in the array
}
}
}
}
// Input values for 'n' and 's'
cout << "Input n and s: ";
cin >> n >> s;
// Output the number of combinations for given 'n' and 's'
cout << "\nNumber of combinations: ";
cout << dp[n][s] << endl;
return 0;
}
Sample Output:
Input n and s: 3 6 Number of combination: 3
Flowchart:
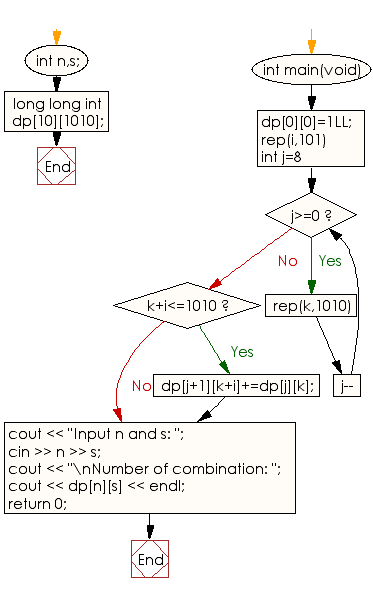
For more Practice: Solve these Related Problems:
- Write a C++ program to determine the number of unique combinations of n distinct numbers that add up to a given sum using recursion.
- Write a C++ program that accepts n numbers and a target sum, then calculates the number of combinations where no number is reused.
- Write a C++ program to find and print all unique combinations of n numbers that sum up to a specified value using backtracking.
- Write a C++ program that uses dynamic programming to count the number of ways n distinct numbers can sum to a target value.
C++ Code Editor:
Previous: Write a C++ program to display all the leap years between two given years. If there is no leap year in the given period,display a suitable message.
Next: Write a C++ program to which replace all the words "dog" with "cat".
What is the difficulty level of this exercise?