C++ Exercises: Check overflow/underflow during various arithmetical operation
Overflow and Underflow in Arithmetic
Write a C++ program to check overflow/underflow during various arithmetic operations.
Sample Solution:
C++ Code :
#include <iostream> // Including the input-output stream header file
using namespace std; // Using the standard namespace
int main() // Start of the main function
{
cout << "\n\n Check overflow/underflow during various arithmetical operation :\n"; // Outputting a message to check overflow/underflow
cout << " Range of int is [-2147483648, 2147483647]"<< endl; // Displaying the range of int
cout << "---------------------------------------------------------------------\n"; // Outputting a separator line
int n1 = 2147483647; // Assigning the maximum range of integer to n1
cout <<" Overflow the integer range and set in minimum range : " << n1 + 1 << endl; // Attempting to overflow by adding 1
cout <<" Increasing from its minimum range : " << n1 + 2 << endl; // Trying to increase beyond the maximum limit
cout <<" Product is :" << n1 * n1 << endl; // Calculating the product of n1 with itself
int n2 = -2147483648; // Assigning the minimum range of integer to n2
cout <<" Underflow the range and set in maximum range : " << n2 - 1 << endl; // Attempting to underflow by subtracting 1
cout <<" Decreasing from its maximum range : " << n2 - 2 << endl; // Trying to decrease beyond the minimum limit
cout <<" Product is : " << n2 * n2 << endl; // Calculating the product of n2 with itself
cout << endl; // Outputting a blank line for better readability
return 0; // Returning 0 to indicate successful program execution
} // End of the main function
Sample Output:
Check overflow/underflow during various arithmetical operation : Range of int is [-2147483648, 2147483647] --------------------------------------------------------------------- Overflow the integer range and set in minimum range : -2147483648 Increasing from its minimum range : -2147483647 Product is :1 Underflow the range and set in maximum range : 2147483647 Decreasing from its maximum range : 2147483646 Product is : 0
Flowchart:
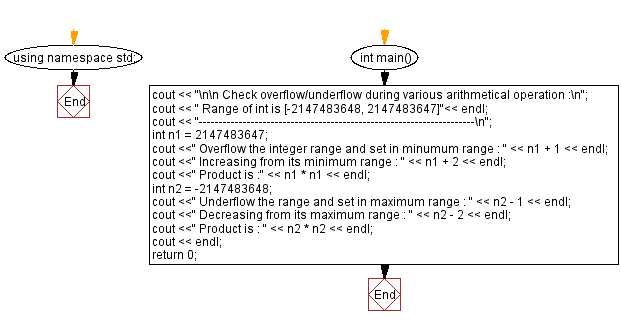
C++ Code Editor:
Previous: Write a program in C++ to display various type or arithmetic operation using mixed data type.
Next: Write a program in C++ to display the operation of pre and post increment and decrement.
What is the difficulty level of this exercise?
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics