C++ Exercises: Display all the leap years between two given years
Leap Years Between Two Years
Write a C++ program to display all the leap years between two given years. If there is no leap year in the given period,display a suitable message.
Note: Range of the two given years: ( 0 < year1 ≤ year2 < 3,000).
Visual Presentation:
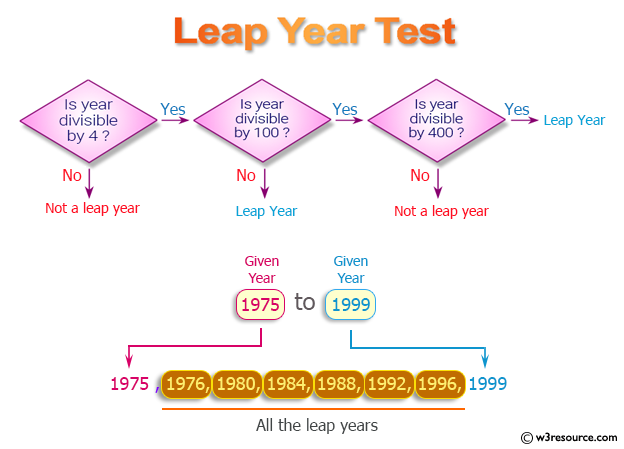
Sample Solution:
C++ Code :
#include <iostream>
// Macros for defining range loops
#define range(i,a,b) for(int (i)=(a);(i)<(b);(i)++)
#define rep(i,n) range(i,0,n)
using namespace std;
// Function to check if a year is a leap year
inline bool isleap(int year) {
if(year % 400 == 0)
return true;
if(year % 100 == 0)
return false;
if(year % 4 == 0)
return true;
return false;
}
int main(void) {
int a, b;
bool space = false;
// Input years
cin >> a >> b;
// Output the range of years provided
cout << "Input years: " << a << " - " << b << endl;
// Output leap years between the given range of years
cout << "\nLeap years between said years:\n";
// Display an empty line if a leap year is found
if(space) puts("");
bool ans = false;
// Loop through the range of years to find leap years
range(i, a, b + 1) {
if(isleap(i)) {
cout << i << endl;
ans = true;
}
}
// Output if no leap years are found within the range
if(!ans) puts("No leap years.");
space = true;
return 0;
}
Sample Output:
Input years: 1975 - 2018 Leap years between said years: 1976 1980 1984 1988 1992 1996 2000 2004 2008 2012 2016
Flowchart:
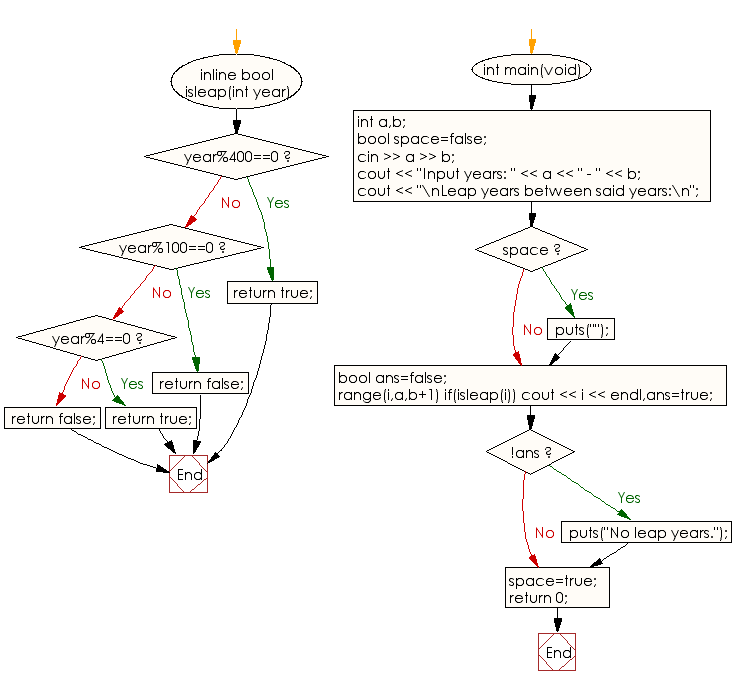
C++ Code Editor:
Previous: Write a C++ program to sum of all positive integers in a sentence.
Next: Write a C++ program that accepts n different numbers (0 to 100) and s which is equal to the sum of the n different numbers.
What is the difficulty level of this exercise?
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics