C++ Exercises: Sum of all positive integers in a sentence
Sum Positive Integers in Text
Write a C++ program to sum all positive integers in a sentence.
Sample string: There are 12 chairs, 15 desks, 1 blackboard and 2 fans.
Output: 30
Sample Solution:
C++ Code :
#include <bits/stdc++.h> // Includes most commonly used headers
using namespace std;
int main() {
string str1; // Declare a string variable to store input
int sum_num = 0, num; // Initialize variables to store the sum of numbers and individual numbers
// Continuously read input lines until EOF
while (getline(cin, str1)) {
// Loop through each character in the string
for (int i = 0; i < (int)str1.size(); i++) {
if (isdigit(str1[i])) continue; // If the character is a digit, continue to the next character
else {
str1[i] = ' '; // Replace non-digit characters with space
}
}
stringstream abc(str1); // Create a stringstream object 'abc' from the modified string
// Extract integers from the stringstream and add them to sum_num
while (abc >> num) {
sum_num += num; // Add each extracted integer to the sum
}
}
// Output the total sum of positive integers
cout << "Sum of all positive integers: " << sum_num << endl;
return 0;
}
Sample Output:
Input number: 12 chairs, 15 desks, 1 blackboard and 2 fans Sum of all positive integers: 30
Flowchart:
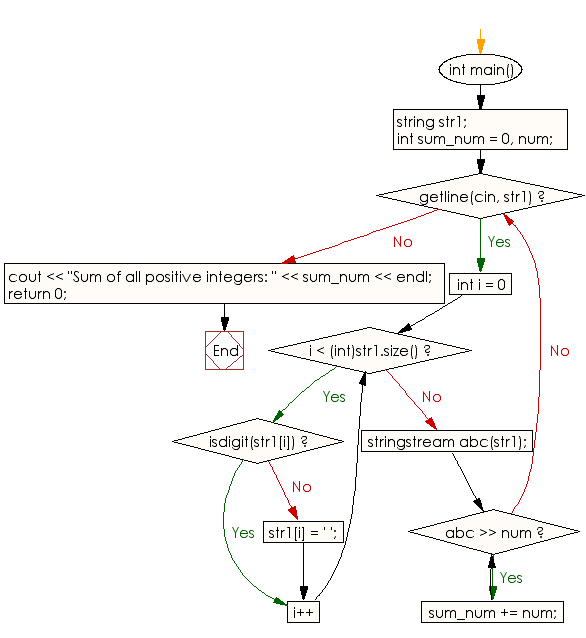
For more Practice: Solve these Related Problems:
- Write a C++ program to parse a sentence, extract all positive integers, and then sum them without using regular expressions.
- Write a C++ program that reads a text string, identifies all numeric substrings, and sums only those that represent positive integers.
- Write a C++ program to scan through a given sentence, convert found numbers to integers, and compute the sum of all positive values.
- Write a C++ program that processes a text containing mixed characters, extracts positive integers, and displays their cumulative sum.
C++ Code Editor:
Previous: Write a C++ program to check whether two straight lines AB and CD are orthogonal or not.
Next: Write a C++ program to display all the leap years between two given years. If there is no leap year in the given period,display a suitable message.
What is the difficulty level of this exercise?