C++ Exercises: Compute total sales amount and the average sales quantity
Sales Total and Average Quantity
Write a C++ program that accepts the sales unit price and sales quantity of various items and computes the total sales amount and the average sales quantity. All input values must be greater than or equal to 0 and less than or equal to 1,000. In addition, the number of pairs of sales unit and sales quantity does not exceed 100. If a fraction occurs in the average of the sales quantity, round to the first decimal place.
Visual Presentation:
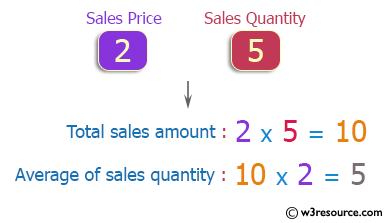
Sample Solution:
C++ Code :
#include <iostream> // Header file for input/output stream operations
using namespace std; // Using the standard namespace
int main()
{
// Declaration of variables
int sale_price, qty, ctr = 0, sum1 = 0, sum2 = 0;
// Prompting user to input Sales Price and Sales Quantity
cout << "Input Sales Price and Sales Quantity: ";
// Loop to read input for sale price and quantity until invalid input
while (cin >> sale_price >> qty)
{
sum1 += sale_price * qty; // Accumulating the total sales amount
sum2 += qty; // Accumulating the total quantity of sales
ctr++; // Incrementing the counter for each input read
}
// Outputting the total sales amount and average sales quantity
cout << "\nTotal of the sales amount and the average of the sales quantity:\n";
cout << sum1 << endl // Outputting total sales amount
<< static_cast<int>(static_cast<double>(sum2) / ctr + 0.5) << endl; // Outputting average sales quantity
return 0; // Indicating successful completion of the program
}
Sample Output:
Sample Input: 2 5 Input Sales Price and Sales Quantity: Total of the sales amount and the average of the sales quantity: 10 5
Flowchart:
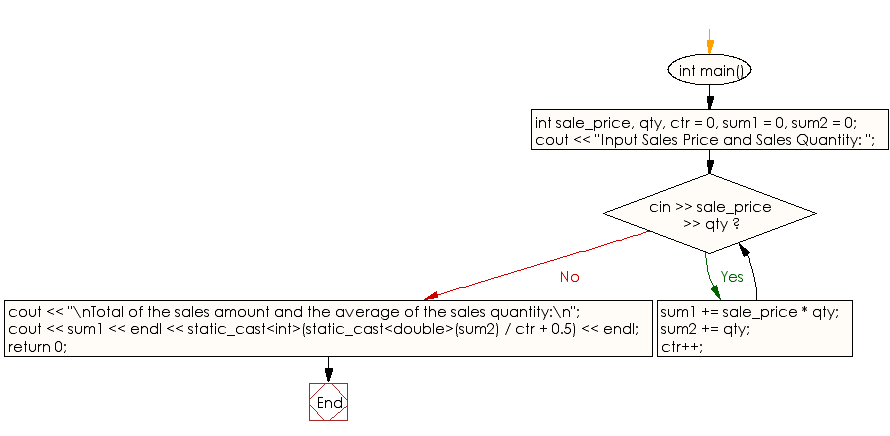
C++ Code Editor:
Previous: Write a C++ program to which reads n digits chosen from 0 to 9 and counts the number of combinations where the sum of the digits equals to given number. Do not use the same digits in a combination.
Next: Write a C++ program that accepts various numbers and compute the difference between the highest number and the lowest number. All input numbers should be real numbers between 0 and 1,000,000. The output (real number) may include an error of 0.01 or less.
What is the difficulty level of this exercise?
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics