C++ Exercises: Reads a sequence of integers and prints mode values of the sequence
Find Mode in Sequence
Write a C++ program that reads a sequence of integers and prints the mode values of the sequence. The number of integers is greater than or equal to 1 and less than or equal to 100.
Note: The mode of a set of data values is the value that appears most often.
Sample Solution:
C++ Code :
#include <iostream> // Including input-output stream header file
#include <vector> // Including vector header file
using namespace std; // Using the standard namespace
int main() {
vector<int> nums(101, 0); // Declaring a vector 'nums' of size 101 initialized with zeros
int n, mode = 0; // Declaring variables 'n' for input number and 'mode' to store the mode value
while (cin >> n) { // Continuously reads integers until the input stream fails
nums[n]++; // Incrementing the count of the corresponding index in the 'nums' vector for the input number 'n'
if (nums[n] > mode) { // Checking if the count of the current number is greater than the mode
mode = nums[n]; // If so, update the mode value
}
}
// Loop through the 'nums' vector to find and print the mode(s)
for (int i = 0; i != nums.size(); ++i) {
if (nums[i] == mode) { // Checking if the count at index 'i' is equal to the mode
cout << i << endl; // If true, print the index (which represents the mode(s))
}
}
return 0; // Indicating successful completion of the program
}
Sample Output:
0 1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100
Flowchart:
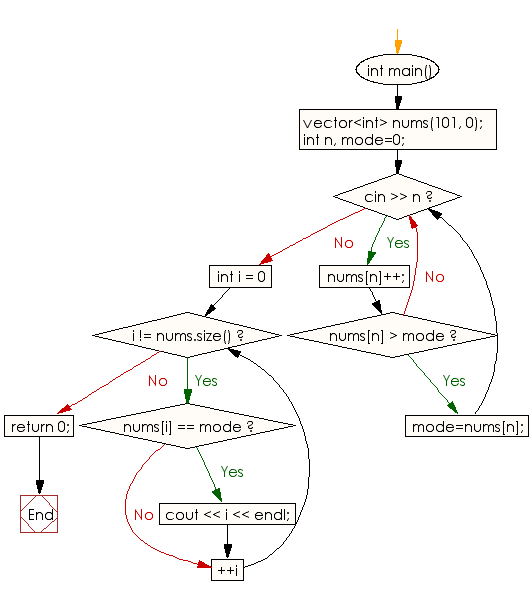
For more Practice: Solve these Related Problems:
- Write a C++ program to determine the mode of a sequence of integers using a hash map and then display all modes in case of ties.
- Write a C++ program that reads a sequence of numbers, computes the frequency of each, and outputs the mode along with its frequency.
- Write a C++ program to find the mode in a sequence of integers without using any STL containers by sorting the array first.
- Write a C++ program that calculates the mode of a sequence, then verifies if the mode occurs more than half the time.
C++ Code Editor:
Previous: Write a C++ program to replace all the lower-case letters of a given string with the corresponding capital letters.
Next: Write a C++ program to which reads n digits chosen from 0 to 9 and counts the number of combinations where the sum of the digits equals to given number. Do not use the same digits in a combination.
What is the difficulty level of this exercise?