C++ Exercises: Replace all the lower-case letters of a given string with the corresponding capital letters
Replace Lowercase with Uppercase
Write a C++ program to replace all the lower-case letters in a given string with the corresponding capital letters.
Visual Presentation:
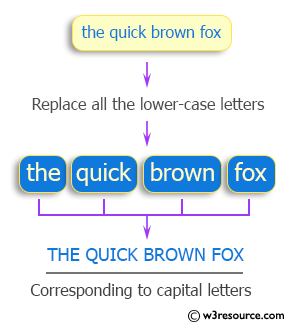
Sample Solution:
C++ Code :
#include <iostream> // Including input-output stream header file
#include <algorithm> // Including algorithm header for transform function
using namespace std; // Using the standard namespace
int main() {
string text; // Declaring a string variable named 'text' to store user input
getline(cin, text); // Getting a line of text input from the user
// Using transform function to convert each character of the string to uppercase
transform(text.begin(), text.end(), text.begin(), ::toupper);
// Displaying the converted string in uppercase
cout << text << endl;
return 0; // Indicating successful completion of the program
}
Sample Output:
Sample Input the quick brown fox jumps over the lazy dog. sample Output THE QUICK BROWN FOX JUMPS OVER THE LAZY DOG.
Flowchart:
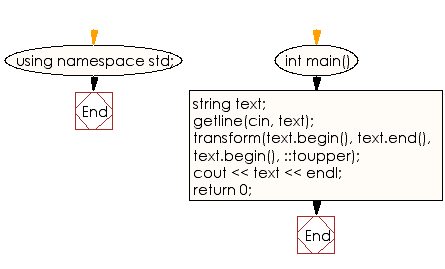
For more Practice: Solve these Related Problems:
- Write a C++ program to convert all lowercase letters in a string to uppercase without using built-in string functions.
- Write a C++ program that reads a sentence and replaces lowercase letters with uppercase using pointer manipulation.
- Write a C++ program to convert a string from lowercase to uppercase and then count the number of changes made.
- Write a C++ program that converts lowercase letters to uppercase by iterating through the ASCII values and using conditional checks.
C++ Code Editor:
Previous: Write a C++ program to read an integer n and prints the factorial of n, assume that n ≤ 10.
Next: Write a C++ program which reads a sequence of integers and prints mode values of the sequence. The number of integers is greater than or equals to 1 and less than or equals to 100.
What is the difficulty level of this exercise?