C++ Exercises: Display various type or arithmetic operation using mixed data type
Mixed Data Types and Arithmetic
Write a C++ program that displays mixed data types and arithmetic operations.
Visual Presentation:
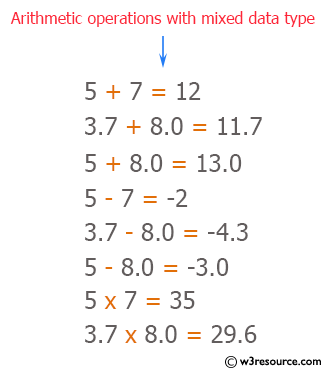
Sample Solution:
C++ Code :
#include <iostream> // Including the input-output stream header file
#include <iomanip> // Including the header file for formatting
using namespace std; // Using the standard namespace
int main() // Start of the main function
{
int m1 = 5, m2 = 7; // Declaring and initializing two integers
double d1 = 3.7, d2 = 8.0; // Declaring and initializing two doubles
cout << "\n\n Display arithmetic operations with mixed data type :\n"; // Outputting a message for arithmetic operations
cout << "---------------------------------------------------------\n"; // Outputting a separator line
cout << fixed << setprecision(1); // Setting floating-point output to fixed with 1 decimal place
// Performing arithmetic operations and displaying results
cout <<" "<< m1 << " + " << m2 << " = " << m1+m2 << endl; // Addition of integers
cout <<" "<< d1 << " + " << d2 << " = " << d1+d2 << endl; // Addition of doubles
cout <<" "<< m1 << " + " << d2 << " = " << m1+d2 << endl; // Addition of an integer and a double
cout <<" "<< m1 << " - " << m2 << " = " << m1-m2 << endl; // Subtraction of integers
cout <<" "<< d1 << " - " << d2 << " = " << d1-d2 << endl; // Subtraction of doubles
cout <<" "<< m1 << " - " << d2 << " = " << m1-d2 << endl; // Subtraction of an integer and a double
cout <<" "<< m1 << " * " << m2 << " = " << m1*m2 << endl; // Multiplication of integers
cout <<" "<< d1 << " * " << d2 << " = " << d1*d2 << endl; // Multiplication of doubles
cout <<" "<< m1 << " * " << d2 << " = " << m1*d2 << endl; // Multiplication of an integer and a double
cout <<" "<< m1 << " / " << m2 << " = " << m1/m2 << endl; // Integer division
cout <<" "<< d1 << " / " << d2 << " = " << d1/d2 << endl; // Division of doubles
cout <<" "<< m1 << " / " << d2 << " = " << m1/d2 << endl; // Division of an integer by a double
cout << endl; // Outputting a blank line for better readability
return 0; // Returning 0 to indicate successful program execution
} // End of the main function
Sample Output:
Display arithmetic operations with mixed data type : --------------------------------------------------------- 5 + 7 = 12 3.7 + 8.0 = 11.7 5 + 8.0 = 13.0 5 - 7 = -2 3.7 - 8.0 = -4.3 5 - 8.0 = -3.0 5 * 7 = 35 3.7 * 8.0 = 29.6 5 * 8.0 = 40.0 5 / 7 = 0 3.7 / 8.0 = 0.5 5 / 8.0 = 0.6
Flowchart:
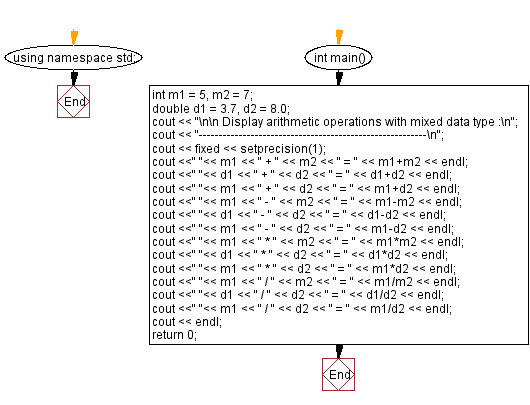
For more Practice: Solve these Related Problems:
- Write a C++ program that mixes integer and floating-point arithmetic to compute a complex expression with implicit type conversions.
- Write a C++ program that performs arithmetic operations on mixed data types and prints the results in scientific notation.
- Write a C++ program to compute arithmetic operations where type casting is explicitly used between mixed data types.
- Write a C++ program that inputs both integer and float values, performs mixed arithmetic operations, and prints a detailed operation log.
C++ Code Editor:
Previous: Write a program in C++ to check whether the primitive values crossing the limits or not.
Next: Write a program in C++ to check overflow/underflow during various arithmetical operation.
What is the difficulty level of this exercise?