C++ Exercises: Prints the central coordinate and the radius of a circumscribed circle of a triangle
Circumscribed Circle of Triangle
Write a C++ program that prints the central coordinate and the radius of a circumscribed circle of a triangle. This circle is created from three points on the plane surface.
Visual Presentation:
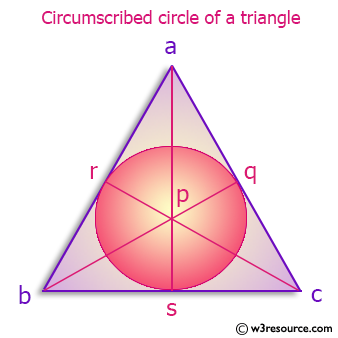
Sample Solution:
C++ Code :
#include <iostream> // Including input-output stream header file
#include <iomanip> // Including input-output manipulator header file
#include <cmath> // Including math functions header file
using namespace std; // Using the standard namespace
int main() {
// Declaring variables for coordinates and calculations
double a, b, c, x1, y1, x2, y2, x3, y3, xp, yp, d, radius;
// Loop to read input coordinates until the end of input
while (cin >> x1 >> y1 >> x2 >> y2 >> x3 >> y3) {
// Calculating lengths of the sides of the triangle formed by the coordinates
a = sqrt((x2 - x1)*(x2 - x1) + (y2 - y1)*(y2 - y1));
b = sqrt((x3 - x1)*(x3 - x1) + (y3 - y1)*(y3 - y1));
c = sqrt((x3 - x2)*(x3 - x2) + (y3 - y2)*(y3 - y2));
// Calculating the radius of the circumscribed circle using triangle sides
radius = (a * b * c) / (sqrt((a + b + c) * (b + c - a) * (c + a - b) * (a + b - c)));
// Calculating the coordinates of the center of the circumscribed circle (x, y)
d = 2 * (x1 * (y2 - y3) + x2 * (y3 - y1) + x3 * (y1 - y2));
xp = ((x1 * x1 + y1 * y1) * (y2 - y3) + (x2 * x2 + y2 * y2) * (y3 - y1) + (x3 * x3 + y3 * y3) * (y1 - y2)) / d;
yp = ((x1 * x1 + y1 * y1) * (x3 - x2) + (x2 * x2 + y2 * y2) * (x1 - x3) + (x3 * x3 + y3 * y3) * (x2 - x1)) / d;
// Outputting the coordinates and radius of the circumscribed circle
cout << fixed << setprecision(3) << "Central coordinate of the circumscribed circle: (" << xp << ", " << yp << ")\nRadius: " << radius << endl;
}
return 0; // Indicating successful completion of the program
}
Sample Output:
Sample input : 6 9 7 Sample Output: 7 9 6
Flowchart:
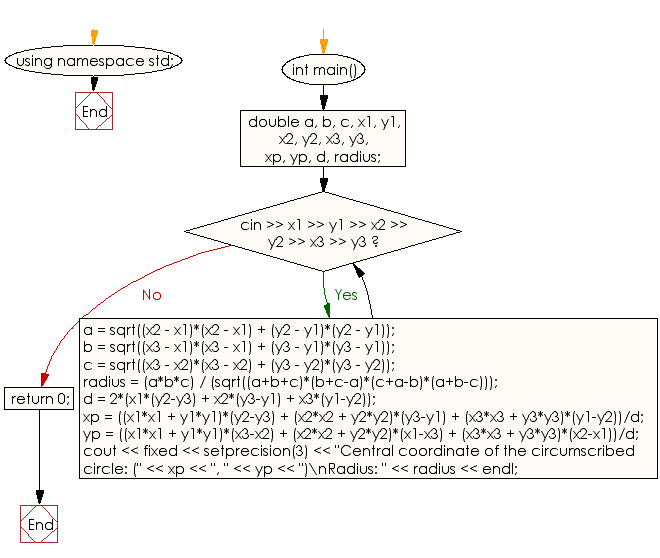
C++ Code Editor:
Previous: Write a C++ program to add all the numbers from 1 to a given number.
Next: Write a C++ program to read seven numbers and sorts them in descending order.
What is the difficulty level of this exercise?
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics