C++ Exercises: Check whether given length of three side form a right triangle
Check Right Triangle
Write a C++ program to check whether a given length of three sides forms a right triangle.
Visual Presentation:
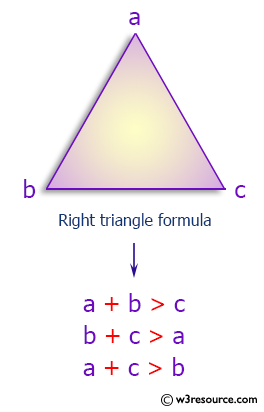
Sample Solution:
C++ Code :
#include <iostream> // Including input-output stream header file
#include <vector> // Including vector header file for vector container
#include <algorithm> // Including algorithm header file for sorting operations
using namespace std; // Using the standard namespace
int main() { // Start of the main function
vector<int> triangle_sides(3); // Creating a vector 'triangle_sides' to store 3 integers (sides of a triangle)
// Inputting 3 integers representing sides of a triangle into the vector
cin >> triangle_sides[0] >> triangle_sides[1] >> triangle_sides[2];
// Sorting the sides of the triangle in ascending order
sort(triangle_sides.begin(), triangle_sides.end());
// Checking if the given sides form a right-angled triangle using Pythagoras theorem
if (triangle_sides[0] * triangle_sides[0] + triangle_sides[1] * triangle_sides[1] == triangle_sides[2] * triangle_sides[2]) {
cout << "Yes" << endl; // If the sides form a right-angled triangle, output "Yes"
} else {
cout << "No" << endl; // If not, output "No"
}
return 0; // Indicating successful completion of the program
}
Sample Output:
Sample input : 6 9 7 Sample Output: No
Flowchart:
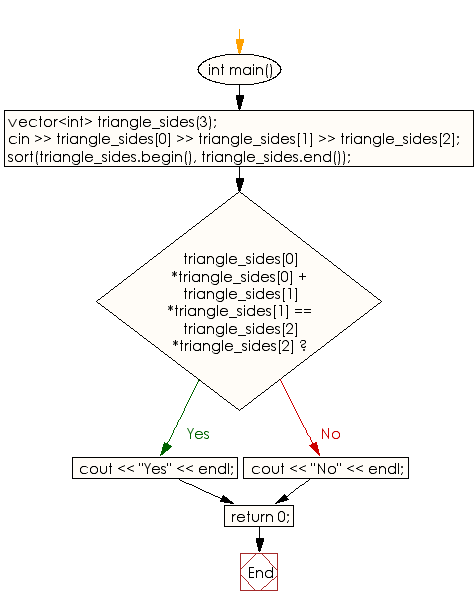
C++ Code Editor:
Previous: Write a C++ program to compute the sum of the two given integers and count the number of digits of the sum value.
Next: Write a C++ program to add all the numbers from 1 to a given number.
What is the difficulty level of this exercise?
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics