C++ Exercises: Compute the sum of the two given integers and count the number of digits of the sum value
Sum of Two Integers and Digit Count
Write a C++ program to compute the sum of the two given integers and count the number of digits in the sum value.
Visual Presentation:
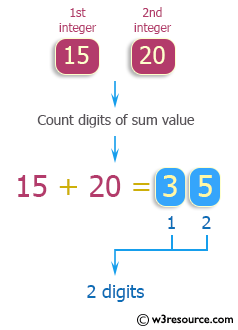
Sample Solution:
C++ Code :
#include <iostream> // Including input-output stream header file
#include <sstream> // Including stringstream header file for string manipulation
using namespace std; // Using the standard namespace
int main() { // Start of the main function
int x, y; // Declaring variables x and y to store integer inputs
while (cin >> x >> y) { // Loop to read integer inputs until the end of input (Ctrl+D in terminal)
stringstream str1; // Declaring a stringstream object 'str1' for string manipulation
str1 << x + y; // Adding integers x and y and storing the result as a string in stringstream 'str1'
cout << str1.str().size() << endl; // Printing the size (number of characters) of the resulting string
}
return 0; // Indicating successful completion of the program
}
Sample Output:
Sample input : 15 20 Sample Output: 2
Flowchart:
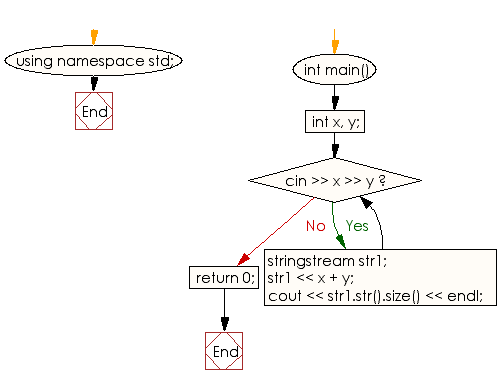
For more Practice: Solve these Related Problems:
- Write a C++ program that computes the sum of two integers and then counts and displays the number of digits in the result using recursion.
- Write a C++ program to add two numbers and count the digits in the sum without converting the number to a string.
- Write a C++ program that calculates the sum of two integers and then prints each digit of the sum on a separate line.
- Write a C++ program to compute the sum of two integers, count the digits in the sum, and then check if the count is a prime number.
C++ Code Editor:
Previous: Write a C++ program which prints three highest numbers from a list of numbers in descending order.
Next: Write a C++ program to check whether given length of three side form a right triangle.
What is the difficulty level of this exercise?