C++ Exercises: Prints three highest numbers from a list of numbers in descending order
Top 3 Highest Numbers
Write a C++ program that prints the three highest numbers from a list of numbers in descending order.
Visual Presentation:
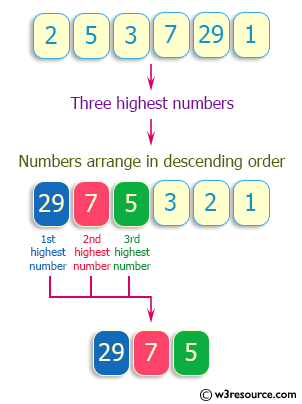
Sample Solution:
C++ Code :
#include <iostream> // Including input-output stream header file
#include <vector> // Including vector header file
#include <algorithm> // Including algorithm header file for sort function
using namespace std; // Using the standard namespace
int main() { // Start of the main function
vector<int> nums; // Declaring a vector to store integers
int n;
while (cin >> n) { // Loop to read integers from input until the end of input (Ctrl+D in terminal)
nums.push_back(n); // Storing the read integers in the vector
}
sort(nums.rbegin(), nums.rend()); // Sorting the vector in descending order
for (int i = 0; i != 3; ++i) { // Looping three times to print the first three elements of the sorted vector
cout << nums[i] << endl; // Printing the elements
}
return 0; // Indicating successful completion of the program
}
Sample Output:
Sample input : 2 5 3 7 29 1 Sample Output: 29 7 5
Flowchart:
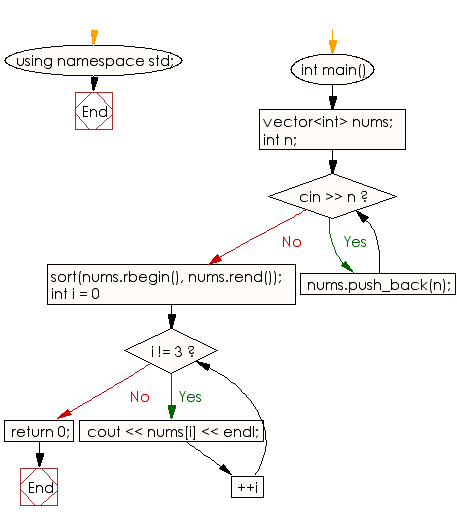
For more Practice: Solve these Related Problems:
- Write a C++ program that reads a list of numbers and prints the three highest unique numbers in descending order.
- Write a C++ program to determine the top 3 highest numbers from an array using sorting and then without sorting.
- Write a C++ program that finds the three largest numbers in a list using a single-pass algorithm and displays them in descending order.
- Write a C++ program to read numbers until a sentinel value is entered and then print the three highest numbers along with their indices.
C++ Code Editor:
Previous: Write a C++ program to which reads an given integer n and prints a twin prime which has the maximum size among twin primes less than or equals to n.
Next: Write a C++ program to compute the sum of the two given integers and count the number of digits of the sum value.
What is the difficulty level of this exercise?