C++ Exercises: Prints a twin prime which has the maximum size among twin primes less than or equals to n
Max Twin Prime Below n
Write a C++ program that reads the integer n and prints a twin prime that has the maximum size among twin primes less than or equal to n.
According to wikipedia "A twin prime is a prime number that is either 2 less or 2 more than another prime number—for example, either member of the twin prime pair (41, 43). In other words, a twin prime is a prime that has a prime gap of two".
Visual Presentation:
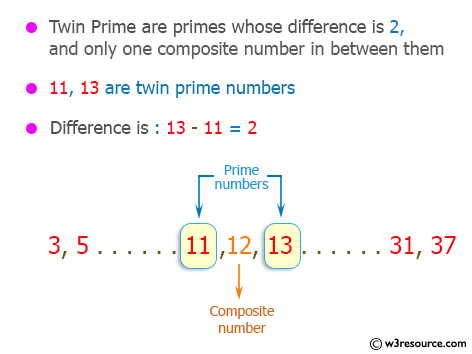
Sample Solution:
C++ Code :
#include <iostream> // Including input-output stream header file
#include <cmath> // Including math functions header file
using namespace std; // Using the standard namespace
int main() { // Start of the main function
const int num_primes = 10005; // Constant to define the maximum limit for finding primes
bool primes[num_primes]; // Declaring an array to store prime flags for numbers up to num_primes
// Initializing the prime number flags to true for all indices except 0 and 1
for (int i = 2; i != num_primes; ++i) {
primes[i] = true;
}
// Loop to sieve out non-prime numbers using the Sieve of Eratosthenes algorithm
for (int i = 2; i != int(sqrt(num_primes)); ++i) {
if (primes[i]) { // Checking if the current number is marked as a prime
// Marking all multiples of the current prime as non-prime
for (int j = 2; i * j < num_primes; ++j) {
primes[i * j] = false;
}
}
}
int n;
cout << "Input an integer:\n"; // Asking the user to input an integer
cin >> n; // Reading the input from the user
cout << "Twin primes are:\n"; // Displaying twin primes
// Loop to find and display twin primes starting from the given input number
for (int i = n; i - 2 >= 0; --i) {
if (primes[i] && primes[i - 2]) { // Checking if the current number and its preceding number are both prime
cout << i - 2 << " " << i << endl; // Displaying the twin primes
break; // Exiting the loop after finding the first twin primes
}
}
return 0; // Indicating successful completion of the program
}
Sample Output:
Input an integer: Twin primes are: 11 13
Flowchart:
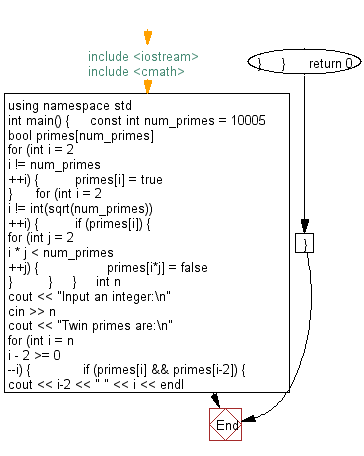
For more Practice: Solve these Related Problems:
- Write a C++ program to find the largest twin prime pair below a given number n using efficient prime checking.
- Write a C++ program that computes all twin prime pairs up to n and then outputs the pair with the maximum sum.
- Write a C++ program to find the twin prime pair below n with the largest difference between its members (should always be 2) and print it.
- Write a C++ program that finds all twin primes below n and then selects the pair with the highest product.
C++ Code Editor:
Previous: Write a C program to swap first and last digits of any number.
Next: Write a C++ program which prints three highest numbers from a list of numbers in descending order.
What is the difficulty level of this exercise?