C++ Exercises: Compute the distance between two points on the surface of earth
Earth Surface Distance
Write a C++ program to compute the distance between two points on the surface of the earth.
Visual Presentation:
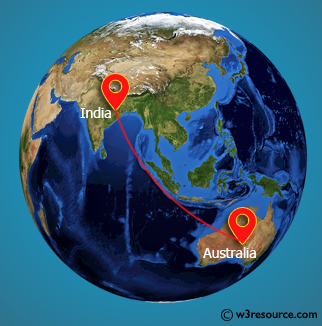
Sample Solution:
C++ Code :
#include <iostream> // Including input-output stream header file
#include <math.h> // Including math functions header file
using namespace std; // Using the standard namespace
int main() { // Start of the main function
double d, la1, la2, lo1, lo2, er, r; // Declaration of variables to store coordinates, distance, and radius
cout << "\n\n Print the distance between two points on the surface of earth:\n"; // Displaying the purpose of the program
cout << "-----------------------------------------------------------------------\n";
cout << " Input the latitude of coordinate 1: "; // Prompting user to input latitude of coordinate 1
cin >> la1; // Taking input from user and storing it in variable 'la1'
cout << " Input the longitude of coordinate 1: "; // Prompting user to input longitude of coordinate 1
cin >> lo1; // Taking input from user and storing it in variable 'lo1'
cout << " Input the latitude of coordinate 2: "; // Prompting user to input latitude of coordinate 2
cin >> la2; // Taking input from user and storing it in variable 'la2'
cout << " Input the longitude of coordinate 2: "; // Prompting user to input longitude of coordinate 2
cin >> lo2; // Taking input from user and storing it in variable 'lo2'
r = 0.01745327; // Conversion factor Pi/180
// Converting latitude and longitude values from degrees to radians
la1 = la1 * r;
la2 = la2 * r;
lo1 = lo1 * r;
lo2 = lo2 * r;
er = 6371.01; // Earth's radius in kilometers
// Calculating the distance between two points on the Earth's surface using Haversine formula
d = er * acos((sin(la1) * sin(la2)) + (cos(la1) * cos(la2) * cos(lo1 - lo2)));
cout << " The distance between those points is: " << d << "\n"; // Displaying the calculated distance
return 0; // Returning 0 to indicate successful program execution
}
Sample Output:
Print the the distance between two points on the surface of earth: ----------------------------------------------------------------------- Input the latitude of coordinate 1: 25 Input the longitude of coordinate 1: 35 Input the latitude of coordinate 2: 35.5 Input the longitude of coordinate 2: 25.5 The distance between those points is: 1480.08
Flowchart:
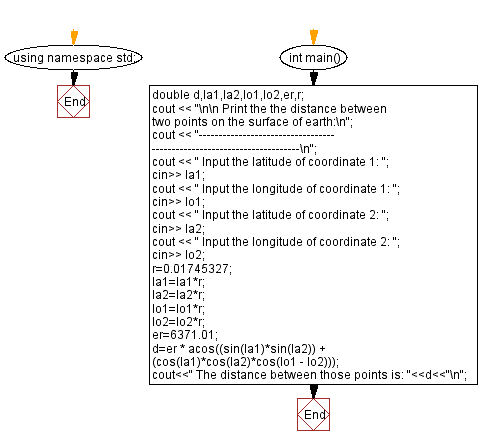
For more Practice: Solve these Related Problems:
- Write a C++ program to calculate the great-circle distance between two points on Earth using the haversine formula.
- Write a C++ program that computes the distance between two geographic coordinates and then outputs the result in kilometers and miles.
- Write a C++ program to determine the distance between two points on Earth and check if they are within a specified travel range.
- Write a C++ program that reads latitude and longitude values, computes the surface distance using spherical geometry, and handles edge cases at the poles.
C++ Code Editor:
Previous: Write a program in C++ to print the area of a polygon.
Next: Write a program in C++ to add two binary numbers.
What is the difficulty level of this exercise?