C++ Exercises: Print the area of a polygon
C++ Basic: Exercise-58 with Solution
Write a C++ program to print the area of a polygon.
Visual Presentation:
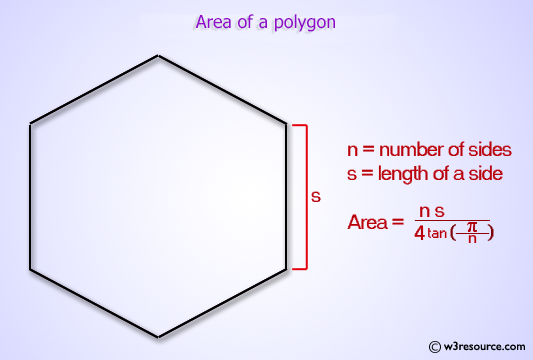
Sample Solution:
C++ Code :
#include <iostream> // Including input-output stream header file
#include <math.h> // Including math functions header file
using namespace std; // Using the standard namespace
int main() { // Start of the main function
float ar, s, n; // Declaration of variables 'ar', 's', and 'n' to store area, side length, and number of sides respectively
cout << "\n\n Print the area of a polygon:\n"; // Displaying the purpose of the program
cout << "---------------------------------\n";
cout << " Input the number of sides of the polygon: "; // Prompting user to input the number of sides
cin >> n; // Taking input from user and storing it in variable 'n'
cout << " Input the length of each side of the polygon: "; // Prompting user to input the side length
cin >> s; // Taking input from user and storing it in variable 's'
// Calculating the area of the polygon using the formula: n * s^2 / (4 * tan(π / n))
ar = (n * (s * s)) / (4.0 * tan((M_PI / n))); // M_PI represents the value of π
cout << " The area of the polygon is: " << ar << "\n"; // Displaying the calculated area
return 0; // Returning 0 to indicate successful program execution
}
Sample Output:
Print the area of a polygon: --------------------------------- Input the number of sides of the polygon: 7 Input the length of each side of the polygon: 6 The area of the ploygon is: 130.821
Flowchart:
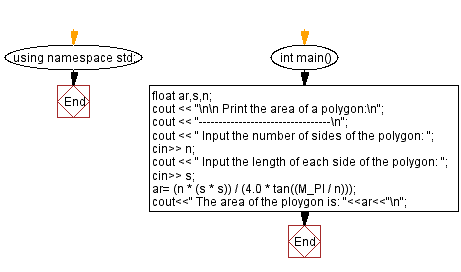
C++ Code Editor:
Previous: Write a program in C++ to print the area of a hexagon.
Next: Write a program in C++ to compute the distance between two points on the surface of earth.
What is the difficulty level of this exercise?
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://w3resource.com/cpp-exercises/basic/cpp-basic-exercise-58.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics