C++ Exercises: Print the area of a hexagon
Hexagon Area Calculation
Write a C++ program to print the area of a hexagon.
Visual Presentation:
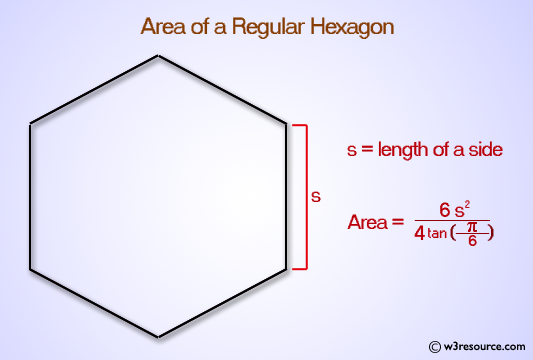
Sample Solution:
C++ Code :
#include <iostream> // Including input-output stream header file
#include <math.h> // Including math functions header file
using namespace std; // Using the standard namespace
int main() { // Start of the main function
float ar, s; // Declaration of variables 'ar' and 's' to store area and side length respectively
cout << "\n\n Print the area of a hexagon:\n"; // Displaying the purpose of the program
cout << "---------------------------------\n";
cout << " Input the side of the hexagon: "; // Prompting user to input side length
cin >> s; // Taking input from user and storing it in variable 's'
// Calculating the area of the hexagon using the formula: 6 * s^2 / (4 * tan(π/6))
ar = (6 * (s * s)) / (4 * tan(M_PI / 6)); // M_PI represents the value of π
cout << "The area of the hexagon is: " << ar << "\n"; // Displaying the calculated area
return 0; // Returning 0 to indicate successful program execution
}
Sample Output:
Print the area of a hexagon: --------------------------------- Input the side of the hexagon: 6 The area of the hexagon is: 93.5307
Flowchart:
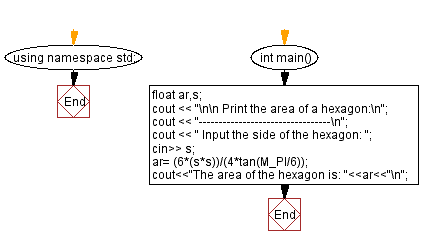
For more Practice: Solve these Related Problems:
- Write a C++ program to calculate the area of a hexagon and then compute the perimeter, given the side length.
- Write a C++ program that calculates the area of a hexagon using trigonometric functions and validates the input for non-negative values.
- Write a C++ program to compute the area of a regular hexagon and then compare it with the area of an inscribed circle.
- Write a C++ program to calculate the area of a hexagon using a user-defined function and display the result with four decimal precision.
C++ Code Editor:
Previous: Write a program in C++ to show the manipulation of a string.
Next: Write a program in C++ to print the area of a polygon.
What is the difficulty level of this exercise?