C++ Exercises: Calculate Compound Interest
Compound Interest Calculation
Write a C++ program to enter P, T, R and calculate compound interest.
Sample Solution:
C++ Code :
#include<iostream> // Including input-output stream header file
#include<math.h> // Including math functions header file
using namespace std; // Using the standard namespace
int main() { // Start of the main function
float p, r, t, cp, ci; // Declaring variables to store principle, rate, time, compound interest, and compound amount
cout << "\n\n Calculate the Compound Interest :\n"; // Displaying the purpose of the program
cout << " -------------------------------------\n";
cout << " Input the Principle: "; // Prompting the user to input the principle amount
cin >> p; // Taking input of the principle amount from the user
cout << " Input the Rate of Interest: "; // Prompting the user to input the rate of interest
cin >> r; // Taking input of the rate of interest from the user
cout << " Input the Time: "; // Prompting the user to input the time period
cin >> t; // Taking input of the time period from the user
ci = p * pow((1 + r / 100), t) - p; // Calculating the compound interest using the formula: P(1 + r/n)^nt - P
cp = p * pow((1 + r / 100), t); // Calculating the compound amount
cout << " The Interest after compounded for the amount " << p << " for " << t << " years @ " << r << " % is: " << ci; // Displaying the calculated compound interest
cout << endl; // Displaying an empty line for better readability
cout << " The total amount after compounded for the amount " << p << " for " << t << " years @ " << r << " % is: " << cp; // Displaying the calculated compound amount
cout << endl; // Displaying an empty line for better readability
cout << endl; // Displaying an empty line for better readability
return 0; // Returning 0 to indicate successful program execution
}
Sample Output:
Calculate the Compound Interest : ------------------------------------- Input the Principle: 20000 Input the Rate of Interest: 10 Input the Time: 1.5 The Interest after compounded for the amount 20000 for 1.5 years @ 10 % is: 3073.8 The total amount after compounded for the amount 20000 for 1.5 years @ 10 % is: 23073.8
Flowchart:
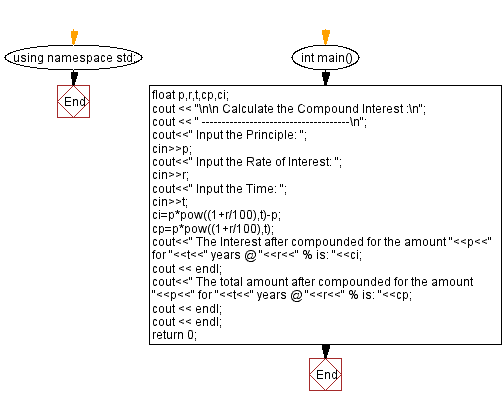
For more Practice: Solve these Related Problems:
- Write a C++ program to compute compound interest with monthly compounding and display both the interest and final amount.
- Write a C++ program that calculates compound interest using a loop for iterative compounding and then compares it to simple interest.
- Write a C++ program to calculate compound interest with user-selectable compounding frequency and format the output with precision.
- Write a C++ program that computes compound interest and then prints a detailed breakdown of the balance after each compounding period.
C++ Code Editor:
Previous: Write a program in C++ to enter P, T, R and calculate Simple Interest.
Next: Write a program in C++ to show the manipulation of a string.
What is the difficulty level of this exercise?