C++ Exercises: Converts kilometers per hour to miles per hour
Kilometers to Miles Conversion
Write a C++ program that converts kilometers per hour to miles per hour.
Visual Presentation:
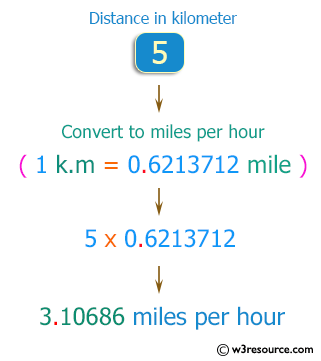
Sample Solution:
C++ Code :
#include<iostream> // Including input-output stream header file
using namespace std; // Using the standard namespace
int main() // Start of the main function
{
float kmph, miph; // Declaring floating-point variables for kilometers per hour and miles per hour
cout << "\n\n Convert kilometers per hour to miles per hour :\n"; // Displaying the purpose of the program
cout << "----------------------------------------------------\n";
cout << " Input the distance in kilometer : "; // Prompting the user to input distance in kilometers
cin >> kmph; // Taking input of distance in kilometers from the user
miph = (kmph * 0.6213712); // Converting kilometers per hour to miles per hour using the conversion factor
// Displaying the converted values
cout << " The " << kmph << " Km./hr. means " << miph << " Miles/hr." << endl;
cout << endl; // Displaying an empty line for better readability
return 0; // Returning 0 to indicate successful program execution
}
Sample Output:
Convert kilometers per hour to miles per hour : ---------------------------------------------------- Input the distance in kilometer : 5 The 5 Km./hr. means 3.10686 Miles/hr.
Flowchart:
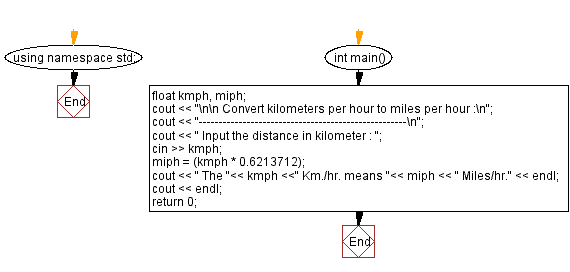
C++ Code Editor:
Previous: Write a program in C++ to enter length in centimeter and convert it into meter and kilometer.
Next: Write a program in C++ to enter two angles of a triangle and find the third angle.
What is the difficulty level of this exercise?
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics