C++ Exercises: Enter length in centimeter and convert it into meter and kilometer
Centimeters to Meters and Kilometers
Write a C++ program to enter length in centimeters and convert it into meters and kilometers.
Visual Presentation:
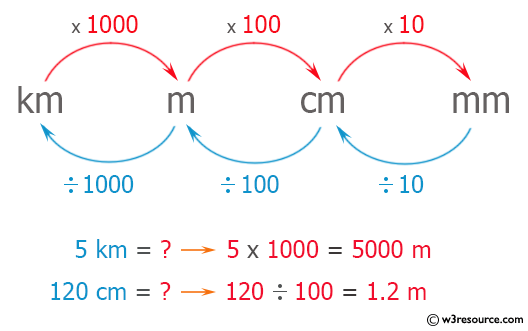
Sample Solution:
C++ Code :
#include<iostream> // Including input-output stream header file
using namespace std; // Using the standard namespace
int main() // Start of the main function
{
float km, met, cent; // Declaring floating-point variables to store distances in kilometers, meters, and centimeters
cout << "\n\n Convert centimeter into meter and kilometer :\n"; // Displaying the purpose of the program
cout << "--------------------------------------------------\n";
cout << " Input the distance in centimeter : "; // Prompting the user to input the distance in centimeters
cin >> cent; // Taking the input of distance in centimeters from the user
met = (cent / 100); // Calculating distance in meters by dividing the input by 100 (1 meter = 100 centimeters)
km = (cent / 100000); // Calculating distance in kilometers by dividing the input by 100000 (1 kilometer = 100,000 centimeters)
cout << " The distance in meter is: " << met << endl; // Displaying the distance in meters
cout << " The distance in kilometer is: " << km << endl; // Displaying the distance in kilometers
cout << endl; // Displaying an empty line for better readability
return 0; // Returning 0 to indicate successful program execution
}
Sample Output:
Convert centimeter into meter and kilometer : -------------------------------------------------- Input the distance in centimeter : 250000 The distance in meter is: 2500 The distance in kilometer is: 2.5
Flowchart:
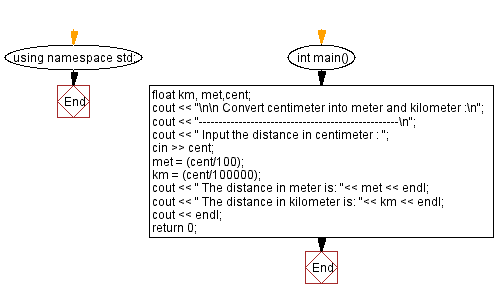
For more Practice: Solve these Related Problems:
- Write a C++ program to convert a distance in centimeters to meters and kilometers, then print the results with appropriate units.
- Write a C++ program that reads a centimeter value, performs conversions to both meters and kilometers, and outputs the results in a formatted table.
- Write a C++ program to convert centimeters to meters and kilometers using functions and validate the input for non-negative values.
- Write a C++ program that accepts a distance in centimeters, converts it to meters and kilometers, and then compares the values to predefined thresholds.
C++ Code Editor:
Previous: Write a program in C++ to print the code (ASCII code / Unicode code etc.) of a given character.
Next: Write a program in C++ that converts kilometers per hour to miles per hour.
What is the difficulty level of this exercise?