C++ Exercises: Swap the values of two variables not using third variable
Swap Variables Without Temp
Write a C++ program that swaps two variables without using a third variable.
Visual Presentation:
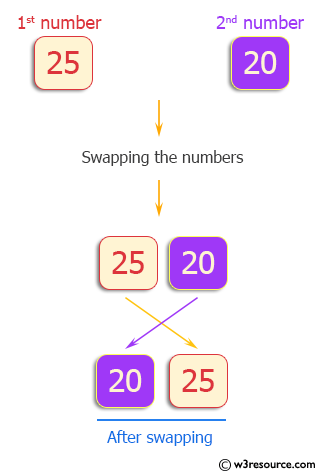
Sample Solution:
C++ Code :
#include <iostream> // Including the input-output stream header file
using namespace std; // Using the standard namespace
int main() // Start of the main function
{
cout << "\n\n Swap two numbers without using third variable:\n"; // Displaying the purpose of the program
cout << "---------------------------------------------------\n";
int num1, num2, temp; // Declaring three integer variables to hold numbers
cout << " Input 1st number : "; // Prompting the user to input the first number
cin >> num1; // Taking the first number as input from the user
cout << " Input 2nd number : "; // Prompting the user to input the second number
cin >> num2; // Taking the second number as input from the user
num2 = num2 + num1; // Swapping logic: adding both numbers and storing the result in num2
num1 = num2 - num1; // Swapping logic: subtracting the original num1 from the updated num2 and storing the result in num1
num2 = num2 - num1; // Swapping logic: subtracting the updated num1 from the updated num2 and storing the result in num2
// Displaying the swapped numbers
cout << " After swapping the 1st number is : " << num1 << "\n";
cout << " After swapping the 2nd number is : " << num2 << "\n\n";
}
Sample Output:
Swap two numbers without using third variable: --------------------------------------------------- Input 1st number : 25 Input 2nd number : 20 After swapping the 1st number is : 20 After swapping the 2nd number is : 25
Flowchart:
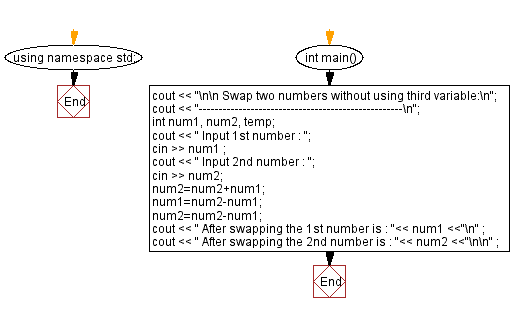
For more Practice: Solve these Related Problems:
- Write a C++ program to swap two numbers without using a third variable by applying bitwise XOR operations.
- Write a C++ program that swaps two integer variables using arithmetic operators and verifies the swap with a printed output.
- Write a C++ program to swap two numbers using a combination of multiplication and division without a temporary variable.
- Write a C++ program that demonstrates swapping of two variables without a temporary variable, then prints both values before and after the swap.
C++ Code Editor:
Previous: Write a program in C++ to calculate the sum of all even and odd numbers in an array.
Next: Write a program in C++ to print the code (ASCII code / Unicode code etc.) of a given character.
What is the difficulty level of this exercise?