C++ Exercises: Calculate the sum of all even and odd numbers in an array
C++ Basic: Exercise-47 with Solution
Write a C++ program to calculate the sum of all even and odd numbers in an array.
Sample Solution:
C++ Code :
#include <iostream> // Including the input-output stream header file
using namespace std; // Using the standard namespace
// Function to separate even and odd numbers and return their sums
int* test(int arr[], int s1) {
static int result[2]; // Static array to store the sum of even and odd numbers
for(int i = 0; i <= s1; i++) // Loop through the array elements
{
// Checking if the current number in the array is even or odd, and updating the sums accordingly
arr[i] % 2 == 0 ? result[0] += arr[i] : result[1] += arr[i];
}
return result; // Returning the array with sums of even and odd numbers
}
int main() // Start of the main function
{
int array1[] = {1, 2, 3, 4, 5, 6, 7, 8}; // Initializing an array
int *eo; // Pointer to hold the result array
int s1 = sizeof(array1) / sizeof(array1[0]); // Calculating the size of the array
// Outputting the original array elements
cout << "Original array: ";
for (int i = 0; i < s1; i++)
cout << array1[i] << " ";
eo = test(array1, s1); // Calling the function to get sums of even and odd numbers
// Outputting the sum of all even and odd numbers
cout << "\nSum of all even and odd numbers: " << *(eo + 0) << "," << *(eo + 1);
return 0; // Return statement indicating successful completion of the program
}
Sample Output:
Original array: 1 2 3 4 5 6 7 8 Sum of all even and odd numbers: 20,16
Flowchart:
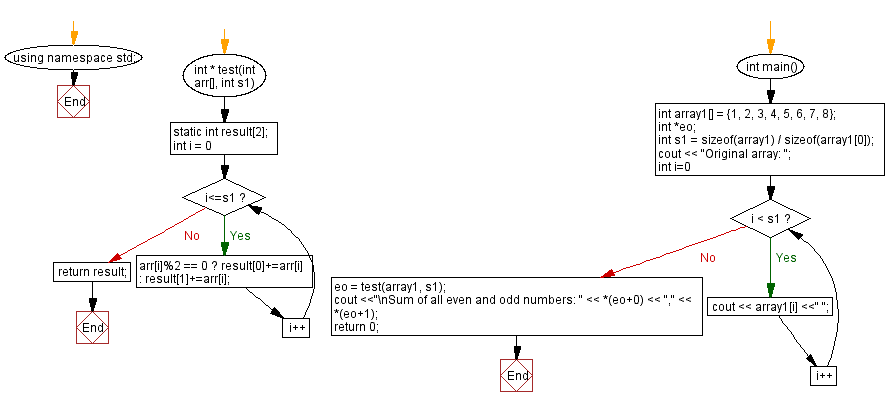
C++ Code Editor:
Previous: Write a program in C++ to calculate the volume of a cylinder.
Next: Write a program in C++ which swap the values of two variables not using third variable.
What is the difficulty level of this exercise?
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://w3resource.com/cpp-exercises/basic/cpp-basic-exercise-47.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics