C++ Exercises: Calculate the volume of a cylinder
Cylinder Volume
Write a C++ program to calculate the volume of a cylinder.
Visual Presentation:
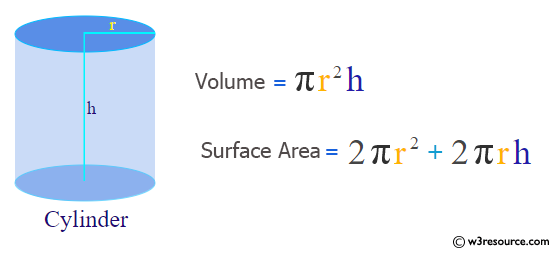
Sample Solution:
C++ Code :
#include <iostream> // Including the input-output stream header file
using namespace std; // Using the standard namespace
int main() // Start of the main function
{
int rad1, hgt; // Declaring variables to store cylinder's radius and height
float volcy; // Declaring variable to store the volume of the cylinder
// Outputting messages to prompt the user to input the radius and height of the cylinder
cout << "\n\n Calculate the volume of a cylinder :\n";
cout << "-----------------------------------------\n";
cout << " Input the radius of the cylinder : ";
cin >> rad1; // Reading the radius input from the user
cout << " Input the height of the cylinder : ";
cin >> hgt; // Reading the height input from the user
// Calculating the volume of the cylinder using the formula: volume = π * radius^2 * height
volcy = (3.14 * rad1 * rad1 * hgt);
// Outputting the calculated volume of the cylinder
cout << " The volume of a cylinder is : " << volcy << endl;
cout << endl;
return 0; // Return statement indicating successful completion of the program
}
Sample Output:
Calculate the volume of a cylinder : ----------------------------------------- Input the radius of the cylinder : 4 Input the height of the cylinder : 8 The volume of a cylinder is : 401.92
Flowchart:
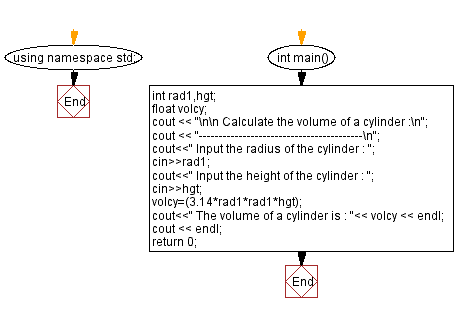
C++ Code Editor:
Previous: Write a program in C++ to calculate the volume of a Cone.
Next: Write a program in C++ to calculate the sum of all even and odd numbers in an array.
What is the difficulty level of this exercise?
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics