C++ Exercises: Calculate the volume of a Cone
Cone Volume
Write a C++ program to calculate the volume of a cone.
Sample Solution:
C++ Code :
#include <iostream> // Including the input-output stream header file
#include <string> // Including the string header file (although it's not used in this code)
using namespace std; // Using the standard namespace
int main() // Start of the main function
{
const float pi = 3.14159; // Declaring a constant variable 'pi' to store the value of pi
float R, H, V; // Declaring variables to store the radius, height, and volume of the cone
cout << "Input Cone's radius: "; // Outputting a message to prompt the user to input the cone's radius
cin >> R; // Reading the cone's radius input from the user
cout << "Input Cone's height: "; // Outputting a message to prompt the user to input the cone's height
cin >> H; // Reading the cone's height input from the user
// Calculating the volume of the cone using the formula: V = (1/3) * π * R^2 * H
V = (1.0 / 3.0) * pi * (R * R) * H;
cout << "The volume of the cone is: " << V; // Outputting the calculated volume of the cone
return 0; // Return statement indicating successful completion of the program
}
Sample Input: 5 3
Sample Output:
Input Cone's radius: Input Cone's height: The volume of the cone is: 78.5397
Flowchart:
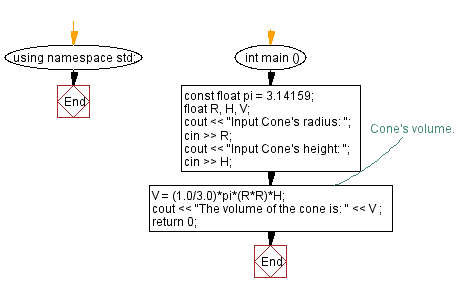
For more Practice: Solve these Related Problems:
- Write a C++ program to compute the volume of a cone given its radius and height, using a function for calculation.
- Write a C++ program that calculates the volume of a cone and compares it to the volume of a cylinder with the same base.
- Write a C++ program to determine the volume of a cone using a constant for pi and display the result with two decimal places.
- Write a C++ program that reads the radius and height of a cone, computes its volume, and then prints the result in a formatted style.
C++ Code Editor:
Previous: Write a language program to get the volume of a sphere with radius 6.
Next: Write a program in C++ to calculate the volume of a cylinder.
What is the difficulty level of this exercise?