C++ Exercises: Get the volume of a sphere with radius 6
Sphere Volume
Write a C++ program to get the volume of a sphere with radius 6.
Visual Presentation:
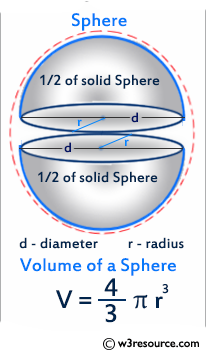
Sample Solution:
C++ Code :
#include <iostream> // Including the input-output stream header file
using namespace std; // Using the standard namespace
int main() // Start of the main function
{
int rad1; // Declaring an integer variable to store the radius of the sphere
float volsp; // Declaring a floating-point variable to store the volume of the sphere
cout << "\n\n Calculate the volume of a sphere :\n"; // Outputting a message indicating the purpose of the program
cout << "---------------------------------------\n"; // Displaying a separator line
cout << " Input the radius of a sphere : "; // Prompting the user to input the radius of the sphere
cin >> rad1; // Reading the radius of the sphere from the user
// Calculating the volume of the sphere using the formula: (4 * π * r^3) / 3
volsp = (4 * 3.14 * rad1 * rad1 * rad1) / 3;
cout << " The volume of a sphere is : " << volsp << endl; // Outputting the calculated volume of the sphere
cout << endl; // Displaying an empty line for better readability
return 0; // Return statement indicating successful completion of the program
}
Sample Output:
Calculate the volume of a sphere : --------------------------------------- Input the radius of a sphere : 5 The volume of a sphere is : 523.333
Flowchart:
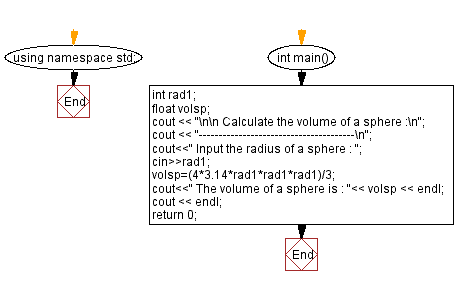
For more Practice: Solve these Related Problems:
- Write a C++ program to calculate the volume of a sphere given its radius with input validation for non-negative values.
- Write a C++ program that computes the volume of a sphere using a user-defined function and then compares it with the volume of a cylinder.
- Write a C++ program to calculate the volume of a sphere and then output the result using fixed-point notation.
- Write a C++ program that accepts the radius from the user, computes the sphere’s volume, and then displays it with a descriptive message.
C++ Code Editor:
Previous: Write a language program which accepts the radius of a circle from the user and compute the area and circumference.
Next: Write a program in C++ to calculate the volume of a Cone.
What is the difficulty level of this exercise?