C++ Exercises: Accepts the user's first and last name and print them in reverse order with a space between them
Reverse First and Last Name
Write a C++ program that accepts the user's first and last name and prints them in reverse order with a space between them.
Visual Presentation:
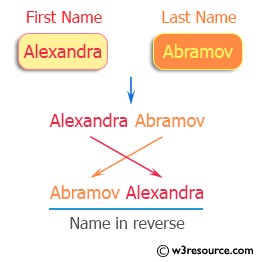
Sample Solution:
C++ Code :
# include <iostream> // Including the input-output stream header file
# include <string> // Including the string header file to work with strings
using namespace std; // Using the standard namespace
int main() // Start of the main function
{
char fname[30], lname[30]; // Declaring character arrays to store first and last names
cout << "\n\n Print the name in reverse where last name comes first:\n"; // Outputting a message indicating the purpose of the program
cout << "-----------------------------------------------------------\n"; // Displaying a separator line
cout << " Input First Name: "; // Prompting the user to input the first name
cin >> fname; // Reading the first name from the user
cout << " Input Last Name: "; // Prompting the user to input the last name
cin >> lname; // Reading the last name from the user
// Outputting the reversed name where the last name comes first
cout << " Name in reverse is: " << lname << " " << fname << endl; // Displaying the reversed name
cout << endl; // Displaying an empty line for better readability
return 0; // Return statement indicating successful completion of the program
}
Sample Output:
Print the name in reverse where last name comes first: ----------------------------------------------------------- Input First Name: Alexandra Input Last Name: Abramov Name in reverse is: Abramov Alexandra
Flowchart:
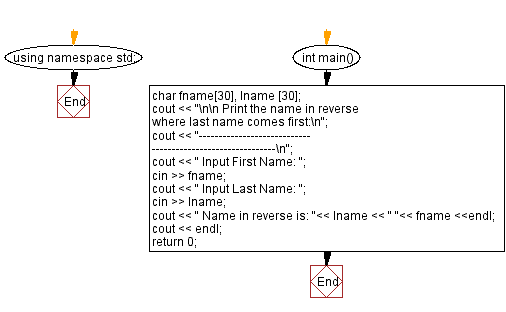
For more Practice: Solve these Related Problems:
- Write a C++ program that accepts a full name, splits it into first and last names, and then prints them in reverse order.
- Write a C++ program to reverse the order of first and last names entered by the user, ensuring proper spacing.
- Write a C++ program that reads the first and last names separately and then outputs them in reverse with a delimiter.
- Write a C++ program that accepts a full name, extracts the first and last name using string manipulation, and then prints them reversed.
C++ Code Editor:
Previous: Write a program in C++ to print an American flag on the screen.
Next: Write a language program which accepts the radius of a circle from the user and compute the area and circumference.
What is the difficulty level of this exercise?