C++ Exercises: Print an American flag on the screen
C++ Basic: Exercise-41 with Solution
Write a C++ program to print an American flag on the screen.
Visual Presentation:
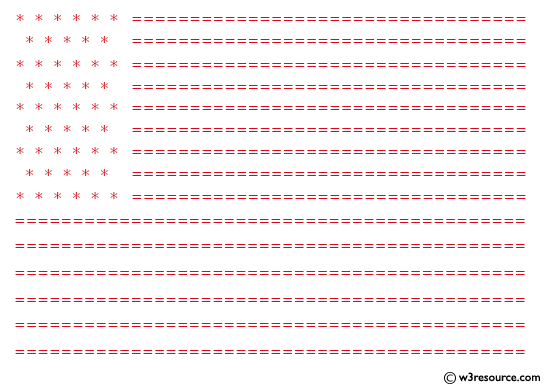
Sample Solution:
C++ Code :
#include <iostream> // Including the input-output stream header file
using namespace std; // Using the standard namespace
int main() // Start of the main function
{
cout << "\n\n Print the American flag:\n"; // Outputting a message indicating the purpose of the program
cout << "-----------------------------\n"; // Displaying a separator line
// Outputting the pattern to represent an American flag with asterisks and equal signs
cout << "* * * * * * ==================================\n"; // First row of the flag
cout << " * * * * * ==================================\n"; // Second row of the flag
cout << "* * * * * * ==================================\n"; // Third row of the flag
cout << " * * * * * ==================================\n"; // Fourth row of the flag
cout << "* * * * * * ==================================\n"; // Fifth row of the flag
cout << " * * * * * ==================================\n"; // Sixth row of the flag
cout << "* * * * * * ==================================\n"; // Seventh row of the flag
cout << " * * * * * ==================================\n"; // Eighth row of the flag
cout << "* * * * * * ==================================\n"; // Ninth row of the flag
// Outputting the lower part of the flag representing the stripes
cout << "==============================================\n"; // Rows representing the stripes of the flag (bottom)
cout << "==============================================\n";
cout << "==============================================\n";
cout << "==============================================\n";
cout << "==============================================\n";
cout << "==============================================\n";
cout << "==============================================\n";
return 0; // Return statement indicating successful completion of the program
}
Sample Output:
Print the American flag: ----------------------------- * * * * * * ================================== * * * * * ================================== * * * * * * ================================== * * * * * ================================== * * * * * * ================================== * * * * * ================================== * * * * * * ================================== * * * * * ================================== * * * * * * ================================== ============================================== ============================================== ============================================== ============================================== ============================================== ==============================================
Flowchart:
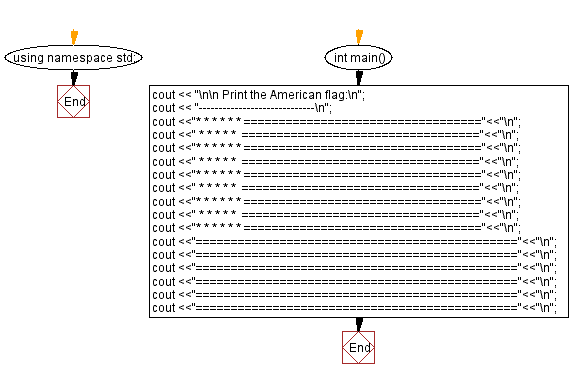
C++ Code Editor:
Previous: Write a program in C++ to print the area and perimeter of a rectangle.
Next: Write a language program in C++ which accepts the user's first and last name and print them in reverse order with a space between them.
What is the difficulty level of this exercise?
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://w3resource.com/cpp-exercises/basic/cpp-basic-exercise-41.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics