C++ Exercises: Print the area and perimeter of a rectangle
Area and Perimeter of Rectangle
Write a C++ program to print the area and perimeter of a rectangle.
Visual Presentation:
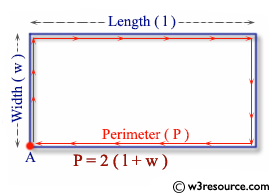
Sample Solution:
C++ Code :
#include <iostream> // Including the input-output stream header file
using namespace std; // Using the standard namespace
int main() // Start of the main function
{
float ar, peri, width, height = 0; // Declaration of variables for area, perimeter, width, and height with initialization
cout << "\n\n Print the area and perimeter of a rectangle:\n"; // Outputting a message indicating the purpose of the program
cout << "----------------------------------------------\n";
cout << " Input the width of the rectangle: "; // Prompting the user to input the width of the rectangle
cin >> width; // Taking user input for the width of the rectangle
cout << " Input the height of the rectangle: "; // Prompting the user to input the height of the rectangle
cin >> height; // Taking user input for the height of the rectangle
peri = 2 * (width + height); // Calculating the perimeter of the rectangle
ar = height * width; // Calculating the area of the rectangle
cout << "The area of the rectangle is: " << ar << "\n"; // Outputting the calculated area of the rectangle
cout << "The perimeter of the rectangle is: " << peri << "\n"; // Outputting the calculated perimeter of the rectangle
}
Sample Output:
Print the area and perimeter of a rectangle: ---------------------------------------------- Input the width of the rectangle: 8.5 Input the height of the rectangle: 5.6 The area of the rectangle is: 47.6 The perimeter of the rectangle is: 28.2
Flowchart:
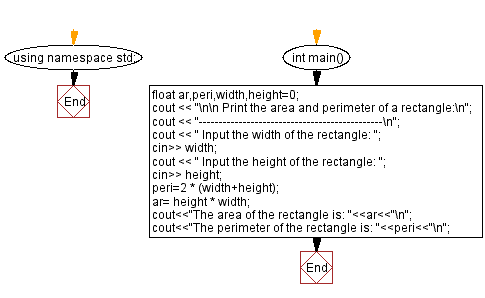
For more Practice: Solve these Related Problems:
- Write a C++ program that calculates the area and perimeter of a rectangle and then computes the ratio between them.
- Write a C++ program to input the dimensions of a rectangle and then display the area and perimeter using formatted output.
- Write a C++ program that reads rectangle dimensions, calculates area and perimeter, and then checks if it is a square.
- Write a C++ program that uses functions to separately compute the area and perimeter of a rectangle and then prints both values.
C++ Code Editor:
Previous: Write a program in C++ to print the specified pattern.
Next: Write a program in C++ to print an American flag on the screen.
What is the difficulty level of this exercise?