C++ Exercises: Print the sum of two numbers using variables
C++ Basic: Exercise-4 with Solution
Write a program in C++ to print the sum of two numbers using variables.
Pictorial Presentation:
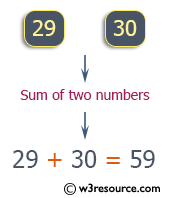
Sample Solution:
C++ Code :
#include <iostream> // Including the input-output stream header file
using namespace std; // Using the standard namespace
int main() // Start of the main function
{
cout << "\n\n Print the sum of two numbers :\n"; // Outputting a message to print the sum of two numbers
cout << "-----------------------------------\n"; // Outputting a separator line
int a; // Declaration of integer variable 'a'
int b; // Declaration of integer variable 'b'
int sum; // Declaration of integer variable 'sum'
a = 29; // Assigning value 29 to variable 'a'
b = 30; // Assigning value 30 to variable 'b'
sum = a + b; // Calculating the sum of 'a' and 'b' and assigning it to 'sum'
cout << " The sum of "<< a << " and "<< b <<" is : "<< sum <<"\n\n" ; // Outputting the calculated sum
return 0; // Returning 0 to indicate successful program execution
} // End of the main function
Sample Output:
Print the sum of two numbers : ----------------------------------- The sum of 29 and 30 is : 59
Flowchart:
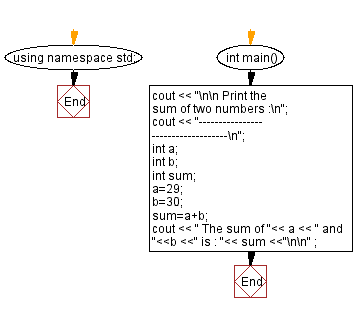
C++ Code Editor:
Previous: Write a program in C++ to find Size of fundamental data types.
Next: Write a program in C++ to check the upper and lower limits of integer.
What is the difficulty level of this exercise?
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics