C++ Exercises: Takes a number as input and prints its multiplication table upto 10
Multiplication Table for Input Number
Write a C++ program that takes a number as input and prints its multiplication table up to 10.
Visual Presentation:
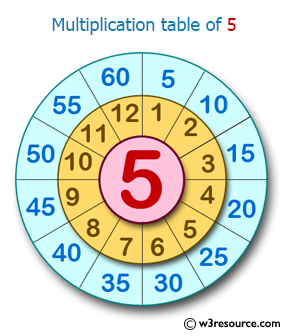
Sample Solution:
C++ Code :
#include <iostream> // Including the input-output stream header file
using namespace std; // Using the standard namespace
int main() // Start of the main function
{
int a, i = 0; // Declaring integer variables 'a' and 'i' with initial values
cout << "\n\n Print the multiplication table of a number up to 10:\n"; // Outputting a message indicating the purpose of the program
cout << "--------------------------------------------------------\n";
cout << " Input a number: ";
cin >> a; // Taking input for variable 'a' from the user
for (i = 1; i <= 10; i++) // Start of a 'for' loop that iterates from 1 to 10
{
cout << a << " x " << i << " = " << a * i << "\n"; // Outputting the multiplication table for the entered number
}
return 0; // Returning 0 to indicate successful program execution
}
Sample Output:
Print the multiplication table of a number upto 10: -------------------------------------------------------- Input a number: 5 5 x 1 = 5 5 x 2 = 10 5 x 3 = 15 5 x 4 = 20 5 x 5 = 25 5 x 6 = 30 5 x 7 = 35 5 x 8 = 40 5 x 9 = 45 5 x 10 = 50
Flowchart:
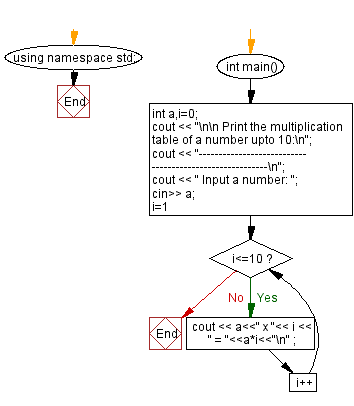
For more Practice: Solve these Related Problems:
- Write a C++ program that prints the multiplication table of a number up to 20, ensuring the table is right-aligned.
- Write a C++ program to generate a multiplication table for a number using nested loops and format the output as a grid.
- Write a C++ program that prints a reversed multiplication table (from 10 down to 1) for an input number.
- Write a C++ program to display the multiplication table of a number and highlight the multiples of 5 with a special marker.
C++ Code Editor:
Previous: Write a program in C++ to print a mystery series from 1 to 50.
Next: Write a program in C++ to print the specified pattern.
What is the difficulty level of this exercise?