C++ Exercises: Print a mystery series from 1 to 50
Print Mystery Series 1 to 50
Write a C++ program to print a mystery series from 1 to 50.
Sample Solution:
C++ Code :
#include <iostream> // Including the input-output stream header file
using namespace std; // Using the standard namespace
int main() // Start of the main function
{
cout << "\n\n Print a mystery series:\n"; // Outputting a message indicating the purpose of the program
cout << "-------------------------\n";
cout << " The series are: \n"; // Outputting a message indicating the series to be printed
int nm1 = 1; // Initializing variable nm1 to 1
while (true) // Starting an infinite loop
{
++nm1; // Incrementing nm1 by 1 in each iteration
if ((nm1 % 3) == 0) // Checking if nm1 is divisible by 3
continue; // Skipping further execution of the loop if nm1 is divisible by 3
if (nm1 == 50) // Checking if nm1 is equal to 50
break; // Exiting the loop if nm1 is equal to 50
if ((nm1 % 2) == 0) // Checking if nm1 is even
{
nm1 += 3; // Adding 3 to nm1 if it's even
}
else
{
nm1 -= 3; // Subtracting 3 from nm1 if it's odd
}
cout << nm1 << " "; // Outputting the current value of nm1 followed by a space
}
cout << endl; // Adding a new line after printing the series
return 0; // Returning 0 to indicate successful program execution
}
Sample Output:
Print a mystery series: ------------------------- The series are: 5 4 2 7 11 10 8 13 17 16 14 19 23 22 20 25 29 28 26 31 35 34 32 37 41 4 0 38 43 47 46 44 49
Flowchart:
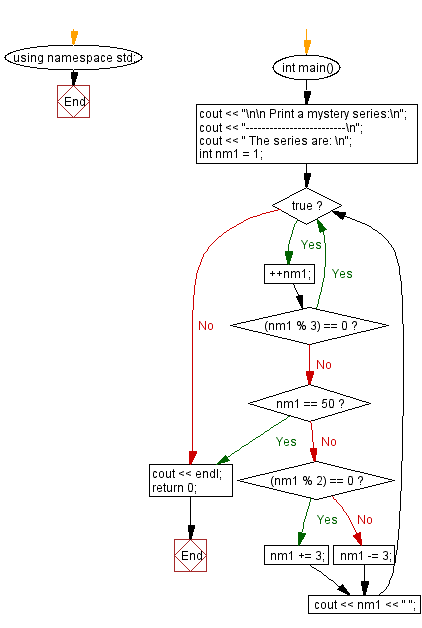
For more Practice: Solve these Related Problems:
- Write a C++ program to generate a mystery series of numbers from 1 to 50 where the pattern alternates between addition and subtraction.
- Write a C++ program that prints a series from 1 to 50 following a hidden pattern based on prime numbers and composite numbers.
- Write a C++ program to output a sequence of numbers from 1 to 50 where every fifth number is replaced by its negative value.
- Write a C++ program that generates a mystery series from 1 to 50 by interweaving two distinct arithmetic progressions.
C++ Code Editor:
Previous: Write a program in C++ to test the Type Casting.
Next: Write a program in C++ that takes a number as input and prints its multiplication table upto 10.
What is the difficulty level of this exercise?