C++ Exercises: Compute the specified expressions and print the output
Compute Specified Expression
Write a program in C++ to compute the specified expressions and print the output.
Sample Solution:
C++ Code:
Sample Output:
Compute the specified expressions and print the output: ------------------------------------------------------------ Result of the expression (25.5 * 3.5 - 3.5 * 3.5) / (40.5 - 4.5) is : 2.13889
Flowchart:
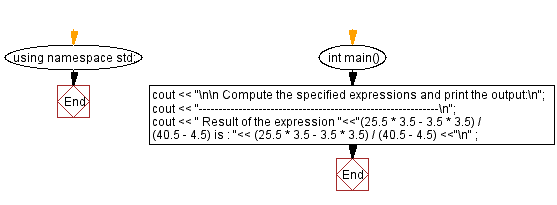
For more Practice: Solve these Related Problems:
- Write a C++ program to compute a given complex arithmetic expression and display each intermediate step.
- Write a C++ program that evaluates multiple expressions and prints their results in a side-by-side comparison.
- Write a C++ program to compute a compound expression with both integer and floating-point operations, ensuring proper type casting.
- Write a C++ program that accepts values from the user to substitute into an expression and then computes and displays the result.
C++ Code Editor:
Previous: Write a C++ program to display the current date and time.
Next: Write a program in C++ to test the Type Casting.
What is the difficulty level of this exercise?