C++ Exercises: Divide two numbers and print on the screen
Divide Two Numbers and Print Quotient
Write a program in C++ to divide two numbers and print them on the screen.
Visual Presentation:
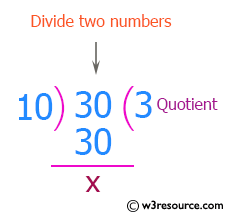
Sample Solution:
C++ Code :
#include <iostream> // Including input-output stream header file
using namespace std; // Using the standard namespace
int main() // Start of the main function
{
cout << "\n\n Divide two numbers and print:\n"; // Outputting a message indicating the purpose of the program
cout << "----------------------------------\n"; // Outputting a separator line
int a; // Declaring an integer variable a
int b; // Declaring an integer variable b
int resdiv; // Declaring an integer variable resdiv to store the result of division
a = 30; // Assigning the value 30 to variable a
b = 10; // Assigning the value 10 to variable b
resdiv = a / b; // Dividing variable a by variable b and storing the result in resdiv
cout << " The quotient of " << a << " and " << b << " is : " << resdiv << "\n\n"; // Outputting the quotient of a and b
return 0; // Returning 0 to indicate successful program execution
}
Sample Output:
Divide two numbers and print: ---------------------------------- The quotient of 30 and 10 is : 3
Flowchart:
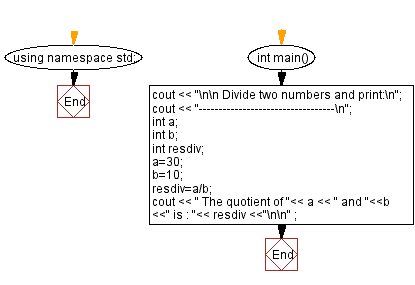
For more Practice: Solve these Related Problems:
- Write a C++ program to divide two numbers entered by the user and print the quotient with error handling for division by zero.
- Write a C++ program that performs division on two integers and prints the quotient as well as the decimal part separately.
- Write a C++ program to divide two numbers using a function and then print the quotient in a formatted output.
- Write a C++ program that takes two numbers, divides them, and then displays the quotient along with a message that explains the division process.
C++ Code Editor:
Previous: Write a program in C++ to check whether a number is positive, negative or zero.
Next: Write a C++ program to display the current date and time.
What is the difficulty level of this exercise?