C++ Exercises: Check whether a number is positive, negative or zero
Check Number Sign (Positive, Negative, Zero)
Write a program in C++ to check whether a number is positive, negative or zero.
Visual Presentation:
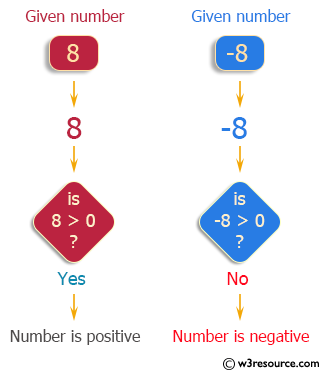
Sample Solution:
C++ Code :
#include <iostream> // Including input-output stream header file
using namespace std; // Using the standard namespace
int main() // Start of the main function
{
signed long num1 = 0; // Declaring and initializing a signed long variable num1 to zero
cout << "\n\n Check whether a number is positive, negative or zero :\n"; // Outputting a message indicating the purpose of the program
cout << "-----------------------------------------------------------\n"; // Outputting a separator line
cout << " Input a number : "; // Prompting the user to input a number
cin >> num1; // Taking input for the variable num1 from the user
if (num1 > 0) // Checking if the entered number is greater than zero
{
cout << " The entered number is positive.\n\n"; // Outputting a message if the number is positive
}
else if (num1 < 0) // Checking if the entered number is less than zero
{
cout << " The entered number is negative.\n\n"; // Outputting a message if the number is negative
}
else // Handling the case where the entered number is zero
{
std::cout << " The number is zero.\n\n"; // Outputting a message if the number is zero
}
return 0; // Returning 0 to indicate successful program execution
} // End of the main function
Sample Output:
Check whether a number is positive, negative or zero : ----------------------------------------------------------- Input a number : 8 The entered number is positive.
Flowchart:
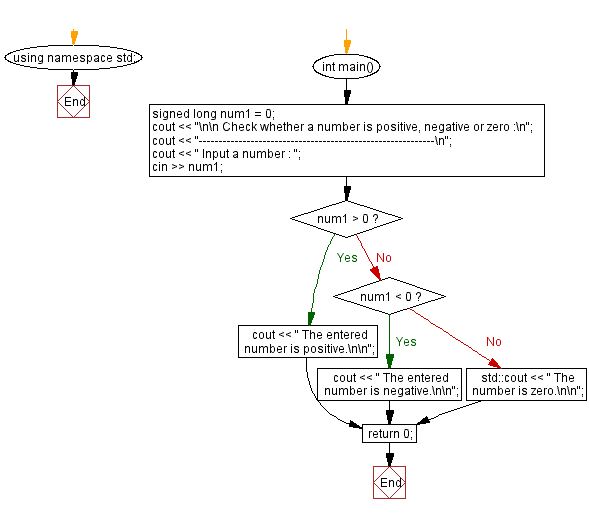
For more Practice: Solve these Related Problems:
- Write a C++ program to determine if a number is positive, negative, or zero and then print a customized message for each case.
- Write a C++ program that reads an integer and prints whether it is positive, negative, or zero using a ternary operator.
- Write a C++ program to check the sign of a number and then display an appropriate ASCII art smiley for positive, frowny for negative, and neutral for zero.
- Write a C++ program that uses a switch statement to classify a number as positive, negative, or zero after comparing it to zero.
C++ Code Editor:
Previous: Write a program in C++ to input a single digit number and print a rectangular form of 4 columns and 6 rows.
Next: Write a program in C++ to divide two numbers and print on the screen.
What is the difficulty level of this exercise?