C++ Exercises: Print a single digit number and print a rectangular form of 4 columns and 6 rows
Rectangular Pattern of Single Digit
Write a C++ program to input a single-digit number and print it in a rectangular form of 4 columns and 6 rows.
Visual Presentation:
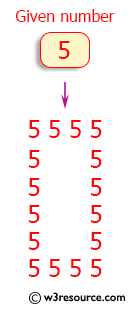
Sample Solution:
C++ Code :
#include <iostream> // Including input-output stream header file
using namespace std; // Using the standard namespace
int main() // Start of the main function
{
int x; // Declaring an integer variable x
cout << "\n\n Make a rectangular shape by a single digit number :\n"; // Outputting a message indicating the purpose of the program
cout << "--------------------------------------------------------\n"; // Outputting a separator line
cout << " Input the number : "; // Prompting the user to input a number
cin >> x; // Taking input for the variable x from the user
// Outputting the rectangular shape using the input number
cout << " " << x << x << x << x << endl; // Outputting a row of the input number repeated four times
cout << " " << x << " " << " " << x << endl; // Outputting a row with the input number separated by spaces
cout << " " << x << " " << " " << x << endl; // Outputting a row with the input number separated by spaces
cout << " " << x << " " << " " << x << endl; // Outputting a row with the input number separated by spaces
cout << " " << x << " " << " " << x << endl; // Outputting a row with the input number separated by spaces
cout << " " << x << x << x << x << endl; // Outputting a row of the input number repeated four times
cout << endl; // Outputting a blank line for better readability
return 0; // Returning 0 to indicate successful program execution
} // End of the main function
Sample Output:
Make a rectangular shape by a single digit number : -------------------------------------------------------- Input the number : 5 5555 5 5 5 5 5 5 5 5 5555
Flowchart:
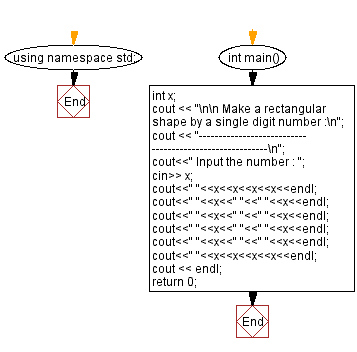
For more Practice: Solve these Related Problems:
- Write a C++ program to print a rectangular pattern using a single digit, but alternate the digit in each row.
- Write a C++ program that inputs a digit and prints a hollow rectangular pattern with that digit as the border.
- Write a C++ program to display a rectangular pattern where the digit appears in a spiral pattern starting from the outer edge.
- Write a C++ program to print a rectangular pattern of a digit, where the first and last rows are filled and the inner rows have the digit only at the borders.
C++ Code Editor:
Previous: Write a program in C++ to compute the total and average of four numbers.
Next: Write a program in C++ to check whether a number is positive, negative or zero.
What is the difficulty level of this exercise?