C++ Exercises: Find Size of fundamental data types
Size of Fundamental Data Types
Write a in C++ program to find the size of fundamental data types.
Sample Solution:
C++ Code :
#include <iostream> // Including the input-output stream header file
using namespace std; // Using the standard namespace
int main() // Start of the main function
{
cout << "\n\n Find Size of fundamental data types :\n"; // Outputting a message for finding the size of fundamental data types
cout << "------------------------------------------\n"; // Outputting a separator line
cout << " The sizeof(char) is : " << sizeof(char) << " bytes \n" ; // Outputting the size of char
cout << " The sizeof(short) is : " << sizeof(short) << " bytes \n" ; // Outputting the size of short
cout << " The sizeof(int) is : " << sizeof(int) << " bytes \n" ; // Outputting the size of int
cout << " The sizeof(long) is : " << sizeof(long) << " bytes \n" ; // Outputting the size of long
cout << " The sizeof(long long) is : " << sizeof(long long) << " bytes \n"; // Outputting the size of long long
cout << " The sizeof(float) is : " << sizeof(float) << " bytes \n" ; // Outputting the size of float
cout << " The sizeof(double) is : " << sizeof(double) << " bytes \n"; // Outputting the size of double
cout << " The sizeof(long double) is : " << sizeof(long double) << " bytes \n"; // Outputting the size of long double
cout << " The sizeof(bool) is : " << sizeof(bool) << " bytes \n\n"; // Outputting the size of bool
return 0; // Returning 0 to indicate successful program execution
} // End of the main function
Sample Output:
Find Size of fundamental data types : ------------------------------------------ The sizeof(char) is : 1 bytes The sizeof(short) is : 2 bytes The sizeof(int) is : 4 bytes The sizeof(long) is : 8 bytes The sizeof(long long) is : 8 bytes The sizeof(float) is : 4 bytes The sizeof(double) is : 8 bytes The sizeof(long double) is : 16 bytes The sizeof(bool) is : 1 bytes
Flowchart:
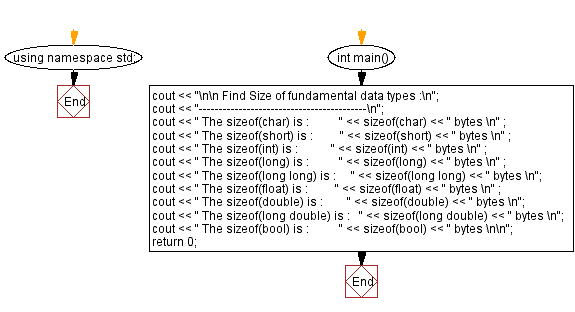
For more Practice: Solve these Related Problems:
- Write a C++ program to print the sizes of various data types using the sizeof operator in a formatted table.
- Write a C++ program that determines the size of fundamental data types and compares them against expected standard sizes.
- Write a C++ program to display the size in bytes of both fundamental and derived data types using typeid.
- Write a C++ program to compute and print the total memory footprint of a structure containing multiple fundamental types.
C++ Code Editor:
Previous: Write a program in C++ to print the sum of two numbers.
Next: Write a program in C++ to print the sum of two numbers using variables.
What is the difficulty level of this exercise?