C++ Exercises: Compute quotient and remainder
Quotient and Remainder Calculation
Write a C++ program to compute the quotient and remainder.
Visual Presentation:
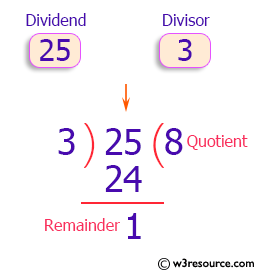
Sample Solution:
C++ Code :
#include <iostream> // Including input-output stream header file
using namespace std; // Using the standard namespace
int main() // Start of the main function
{
int dividend, divisor, quotient, remainder; // Declaring integer variables for dividend, divisor, quotient, and remainder
cout << "\n\n Compute quotient and remainder :\n"; // Outputting a message indicating the purpose of the program
cout << "-------------------------------------\n"; // Outputting a separator line
cout << " Input the dividend : "; // Prompting the user to input the dividend
cin >> dividend; // Taking input for the dividend from the user
cout << " Input the divisor : "; // Prompting the user to input the divisor
cin >> divisor; // Taking input for the divisor from the user
quotient = dividend / divisor; // Calculating the quotient of the division
remainder = dividend % divisor; // Calculating the remainder of the division
cout << " The quotient of the division is : " << quotient << endl; // Displaying the calculated quotient
cout << " The remainder of the division is : " << remainder << endl; // Displaying the calculated remainder
cout << endl; // Outputting a blank line for better readability
return 0; // Returning 0 to indicate successful program execution
} // End of the main function
Sample Output:
Compute quotient and remainder : ------------------------------------- Input the dividend : 25 Input the divisor : 3 The quotient of the division is : 8 The remainder of the division is : 1
Flowchart:
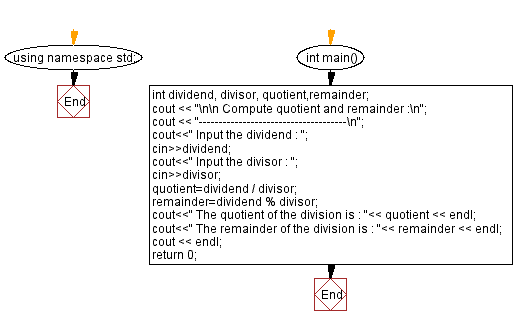
For more Practice: Solve these Related Problems:
- Write a C++ program to compute the quotient and remainder of two numbers and display them with descriptive labels.
- Write a C++ program that reads two integers and computes both the division result and the modulo result without using built-in operators.
- Write a C++ program to calculate the quotient and remainder and then use the results in a subsequent arithmetic expression.
- Write a C++ program that takes two user inputs, computes the quotient and remainder, and then prints a formatted message explaining the division process.
C++ Code Editor:
Previous: Write a program in C++ to find the area of Scalene Triangle.
Next: Write a program in C++ to compute the total and average of four numbers.
What is the difficulty level of this exercise?