C++ Exercises: Find the area of Scalene Triangle
Scalene Triangle Area Calculation
Write a C++ program to find the area of the Scalene Triangle.
Visual Presentation:
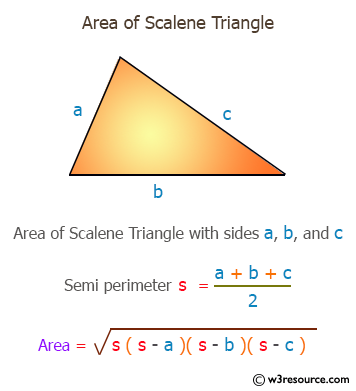
Sample Solution:
C++ Code :
#include <iostream> // Including the input-output stream header file
#include <math.h> // Including the math library for mathematical functions
#define PI 3.14159 // Defining the value of PI as a constant
using namespace std; // Using the standard namespace
int main() // Start of the main function
{
float side1, side2, ang1, area; // Declaring floating-point variables for sides, angle, and area
cout << "\n\n Find the area of Scalene Triangle :\n"; // Outputting a message indicating the purpose of the program
cout << "----------------------------------------\n"; // Outputting a separator line
cout << " Input the length of a side of the triangle : "; // Prompting the user to input the length of one side of the triangle
cin >> side1; // Taking input for the length of one side from the user
cout << " Input the length of another side of the triangle : "; // Prompting the user to input the length of another side of the triangle
cin >> side2; // Taking input for the length of another side from the user
cout << " Input the angle between these sides of the triangle : "; // Prompting the user to input the angle between the given sides
cin >> ang1; // Taking input for the angle from the user
// Calculating the area of the Scalene Triangle using the formula: (side1 * side2 * sin(angle))/2
area = (side1 * side2 * sin((PI / 180) * ang1)) / 2;
cout << " The area of the Scalene Triangle is : " << area << endl; // Displaying the calculated area of the triangle
cout << endl; // Outputting a blank line for better readability
return 0; // Returning 0 to indicate successful program execution
} // End of the main function
Sample Output:
Find the area of Scalene Triangle : ---------------------------------------- Input the length of a side of the triangle : 5 Input the length of another side of the triangle : 6 Input the angle between these sides of the triangle : 6 The area of the Scalene Triangle is : 1.56793
Flowchart:
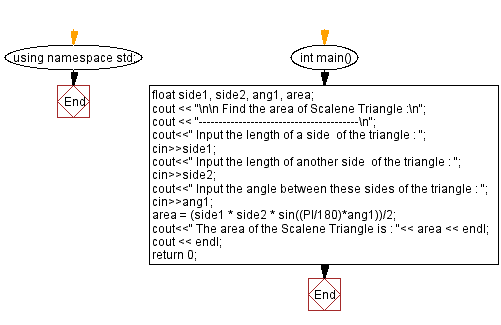
For more Practice: Solve these Related Problems:
- Write a C++ program to compute the area of a scalene triangle given two sides and the included angle using the sine formula.
- Write a C++ program that calculates the area of a scalene triangle by first determining the semi-perimeter and then applying Heron’s formula.
- Write a C++ program to compute the area of a scalene triangle with user-defined functions and validate the triangle inequality.
- Write a C++ program that inputs three sides of a triangle, verifies if it is scalene, and then calculates its area using appropriate formulas.
C++ Code Editor:
Previous: Write a program in C++ to convert temperature in Celsius to Kelvin.
Next: Write a program in C++ to compute quotient and remainder.
What is the difficulty level of this exercise?