C++ Exercises: Find the third angle of a triangle
Find Third Angle of Triangle
Write a C++ program to find the third angle of a triangle.
Visual Presentation:
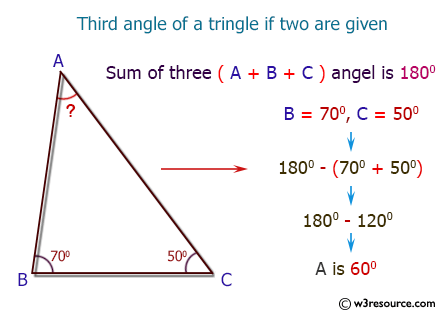
Sample Solution:
C++ Code :
#include <iostream> // Including the input-output stream header file
using namespace std; // Using the standard namespace
int main() // Start of the main function
{
float ang1, ang2, ang3; // Declaring floating-point variables for angles of the triangle
cout << "\n\n Find the third angle of a triangle :\n"; // Outputting a message indicating calculation of the third angle
cout << "-----------------------------------------\n"; // Outputting a separator line
cout << " Input the 1st angle of the triangle : "; // Prompting the user to input the 1st angle of the triangle
cin >> ang1; // Taking input for the 1st angle from the user
cout << " Input the 2nd angle of the triangle : "; // Prompting the user to input the 2nd angle of the triangle
cin >> ang2; // Taking input for the 2nd angle from the user
ang3 = 180 - (ang1 + ang2); // Calculating the third angle of the triangle: 180 - (sum of the 1st and 2nd angles)
cout << " The 3rd of the triangle is : " << ang3 << endl; // Displaying the calculated third angle of the triangle
cout << endl; // Outputting a blank line for better readability
return 0; // Returning 0 to indicate successful program execution
} // End of the main function
Sample Output:
Find the third angle of a triangle : ----------------------------------------- Input the 1st angle of the triangle : 30 Input the 2nd angle of the triangle : 60 The 3rd of the triangle is : 90
Flowchart:
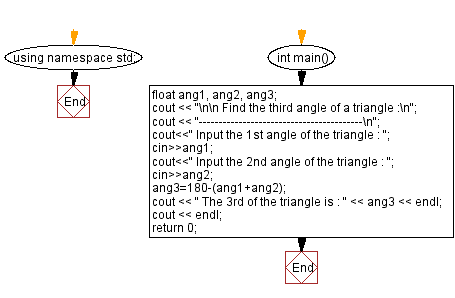
For more Practice: Solve these Related Problems:
- Write a C++ program to compute the third angle of a triangle after validating that the sum of the first two angles is less than 180°.
- Write a C++ program that calculates the missing angle of a triangle and prints an error if the input angles are invalid.
- Write a C++ program to determine the third angle of a triangle using user-defined functions and proper input validation.
- Write a C++ program that reads two angles, computes the third angle, and then categorizes the triangle based on its angles.
C++ Code Editor:
Previous: Write a program in C++ to convert temperature in Fahrenheit to Celsius.
Next: Write a program in C++ that converts kilometers per hour to miles per hour.
What is the difficulty level of this exercise?