C++ Exercises: Find the area and circumference of a circle
Area and Circumference of Circle
Write a C++ program to find the area and circumference of a circle.
Visual Presentation:
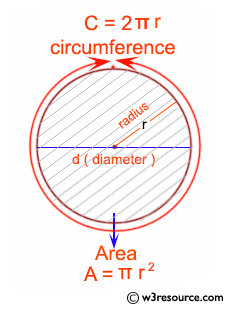
Sample Solution:
C++ Code :
#include <iostream> // Including the input-output stream header file
#define PI 3.14159 // Defining the constant value of PI
using namespace std; // Using the standard namespace
int main() // Start of the main function
{
float radius, area, circum; // Declaring floating-point variables for radius, area, and circumference
cout << "\n\n Find the area and circumference of any circle :\n"; // Outputting a message indicating the calculation of circle area and circumference
cout << "----------------------------------------------------\n"; // Outputting a separator line
cout << " Input the radius(1/2 of diameter) of a circle : "; // Prompting the user to input the radius of the circle
cin >> radius; // Taking input for the radius from the user
circum = 2 * PI * radius; // Calculating the circumference of the circle: 2 * PI * radius
area = PI * (radius * radius); // Calculating the area of the circle: PI * radius^2
cout << " The area of the circle is : " << area << endl; // Displaying the calculated area of the circle
cout << " The circumference of the circle is : " << circum << endl; // Displaying the calculated circumference of the circle
cout << endl; // Outputting a blank line for better readability
return 0; // Returning 0 to indicate successful program execution
} // End of the main function
Sample Output:
Find the area and circumference of any circle : ---------------------------------------------------- Input the radius(1/2 of diameter) of a circle : 5 The area of the circle is : 78.5397 The circumference of the circle is : 31.4159
Flowchart:
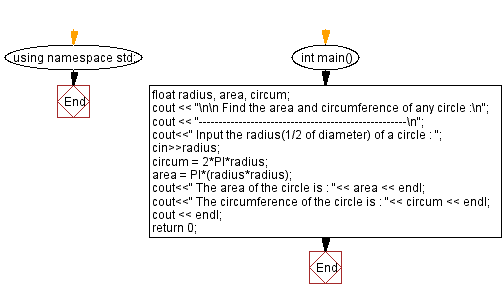
For more Practice: Solve these Related Problems:
- Write a C++ program to compute the area and circumference of a circle when given the diameter instead of the radius.
- Write a C++ program that calculates the area and circumference of a circle and formats the results to three decimal places.
- Write a C++ program to compute the area and circumference of a circle and then compare them to a fixed reference circle.
- Write a C++ program that calculates the area and circumference of a circle using a function and then prints a summary report.
C++ Code Editor:
Previous: Write a program in C++ to find the area of any triangle using Heron's Formula.
Next: Write a program in C++ to convert temperature in Celsius to Fahrenheit.
What is the difficulty level of this exercise?