C++ Exercises: Calculate the volume of a cube
Volume of a Cube
Write a C++ program that calculates the volume of a cube.
Visual Presentation:
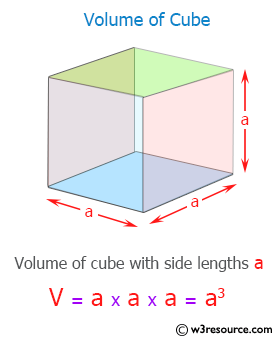
Sample Solution:
C++ Code :
#include <iostream> // Including the input-output stream header file
using namespace std; // Using the standard namespace
int main() // Start of the main function
{
int sid1; // Declaring an integer variable sid1 to store the side length of the cube
float volcu; // Declaring a floating-point variable volcu to store the volume of the cube
cout << "\n\n Calculate the volume of a cube :\n"; // Outputting a message indicating the calculation of cube volume
cout << "---------------------------------------\n"; // Outputting a separator line
cout << " Input the side of a cube : "; // Prompting the user to input the side length of the cube
cin >> sid1; // Taking input for the side length from the user
// Calculating the volume of the cube using the formula: side * side * side
volcu = (sid1 * sid1 * sid1);
cout << " The volume of a cube is : "<< volcu << endl; // Displaying the calculated volume of the cube
cout << endl; // Outputting a blank line for better readability
return 0; // Returning 0 to indicate successful program execution
} // End of the main function
Sample Output:
Calculate the volume of a cube : --------------------------------------- Input the side of a cube : 5 The volume of a cube is : 125
Flowchart:
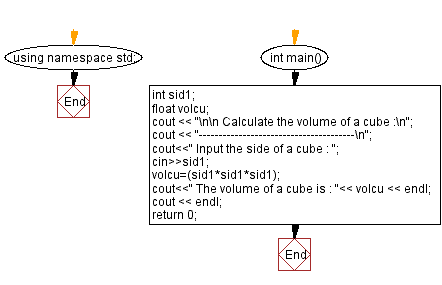
For more Practice: Solve these Related Problems:
- Write a C++ program to calculate the volume of a cube when the edge length is input as a floating-point number.
- Write a C++ program that computes the volume of a cube and then determines the surface area.
- Write a C++ program to calculate the volume of a cube and display both the volume and the length of its space diagonal.
- Write a C++ program that reads the edge length of a cube from the keyboard and prints its volume using a user-defined function.
C++ Code Editor:
Previous: Write a program in C++ to calculate the volume of a sphere.
Next: Write a program in C++ to calculate the volume of a cylinder.
What is the difficulty level of this exercise?