C++ Exercises: Compute the difference between the largest and smallest values in a given array of integers and length one or more
C++ Basic Algorithm: Exercise-99 with Solution
Difference Between Largest and Smallest Values in Array
Write a C++ program to compute the difference between the largest and smallest values in a given array of integers and length one or more.
Sample Solution:
C++ Code :
#include <iostream> // Including input-output stream header file
using namespace std; // Using standard namespace
// Function definition that calculates the difference between the largest and smallest integers in an array
static int test(int nums[], int arr_length)
{
int small_num = 0, biggest_num = 0; // Initializing variables to store smallest and biggest numbers
// Checking if the array has at least one element
if (arr_length > 0)
small_num = biggest_num = nums[0]; // Setting the first element as both the smallest and biggest number initially
// Loop through the array to find the smallest and biggest numbers
for (int i = 1; i < arr_length; i++)
{
small_num = min(small_num, nums[i]); // Updating small_num if a smaller number is found
biggest_num = max(biggest_num, nums[i]); // Updating biggest_num if a larger number is found
}
return biggest_num - small_num; // Returning the difference between the largest and smallest numbers
}
int main()
{
int nums1[] = { 1, 5, 7, 9, 10, 12}; // Initializing array nums1 with various integers
int arr_length = sizeof(nums1) / sizeof(nums1[0]); // Calculating length of array nums1
// Calling test function with nums1 and displaying the difference between largest and smallest numbers
cout << test(nums1, arr_length) << endl;
int nums2[] = {0, 2, 4, 6, 8, 10}; // Initializing array nums2 with even integers
arr_length = sizeof(nums2) / sizeof(nums2[0]); // Calculating length of array nums2
// Calling test function with nums2 and displaying the difference between largest and smallest numbers
cout << test(nums2, arr_length) << endl;
return 0; // Returning 0 to indicate successful completion of the program
}
Sample Output:
11 10
Visual Presentation:
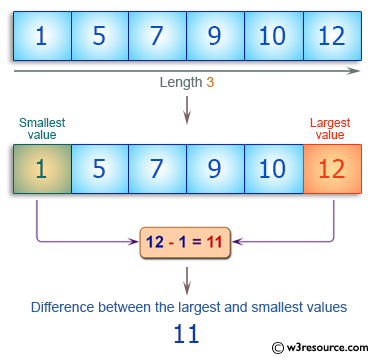
Flowchart:
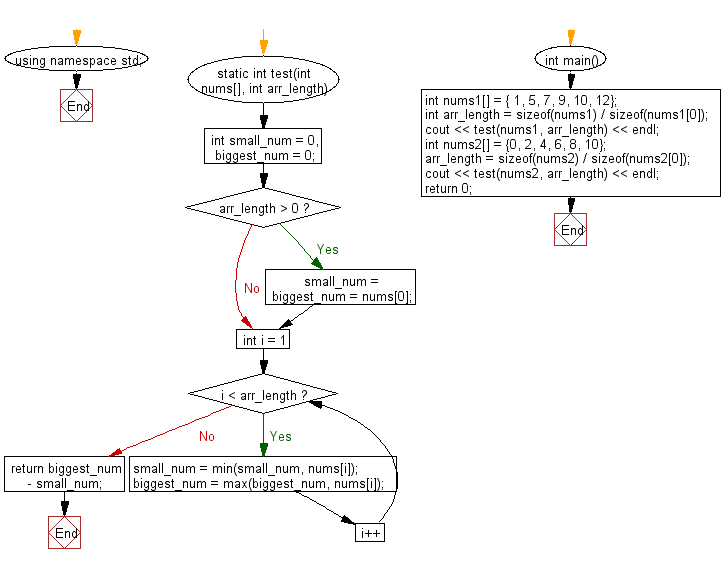
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a C++ program to count even number of elements in a given array of integers.
Next: Write a C++ program to compute the sum of values in a given array of integers except the number 17. Return 0 if the given array has no integer.
What is the difficulty level of this exercise?
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://w3resource.com/cpp-exercises/basic-algorithm/cpp-basic-algorithm-exercise-99.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics