C++ Exercises: Create a new array length 3 from a given array the elements from the middle of the array
Create Array of Middle 3 Elements (Length ≥ 3)
Write a C++ program to create an array length 3 from a given array (length at least 3) using the elements from the middle of the array.
Sample Solution:
C++ Code :
#include <iostream> // Including input-output stream header file
using namespace std; // Using standard namespace
static int* test(int numbers[], int arr_length) // Function definition that creates an array with three elements from the middle of the input array and returns a pointer to it
{
static int result[] { numbers[arr_length / 2 - 1], numbers[arr_length / 2], numbers[arr_length / 2 + 1] }; // Creating a new array 'result' with three elements from the middle of the 'numbers' array
return result; // Returning a pointer to the newly created array
}
int main()
{
int *result, i; // Declaring pointer variable result and integer i
int nums[] = {1, 5, 7, 9, 11, 13}; // Initializing array nums with values
int arr_length = sizeof(nums) / sizeof(nums[0]); // Calculating length of array nums
cout << "Original array:" << endl; // Printing a message
for ( i = 0; i < arr_length; i++ ) // Loop to print elements of the nums array
cout << nums[i] << " ";
result = test(nums, arr_length); // Calling the test function and storing the returned pointer in result
cout << "\nNew array:" << endl; // Printing a message
arr_length = sizeof(result) / sizeof(result[0]); // Calculating the length of array pointed by result (This may not work as intended, see explanation below)
for ( i = 0; i <= arr_length; i++ ) { // Loop to print elements of the array pointed by result
cout << *(result + i) << " "; // Printing elements of the array pointed by result
}
return 0; // Returning 0 to indicate successful completion of the program
}
Sample Output:
Original array: 1 5 7 9 11 13 New array: 7 9 11
Visual Presentation:
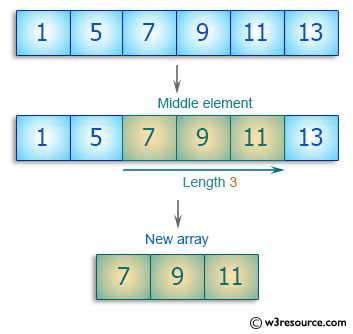
Flowchart:
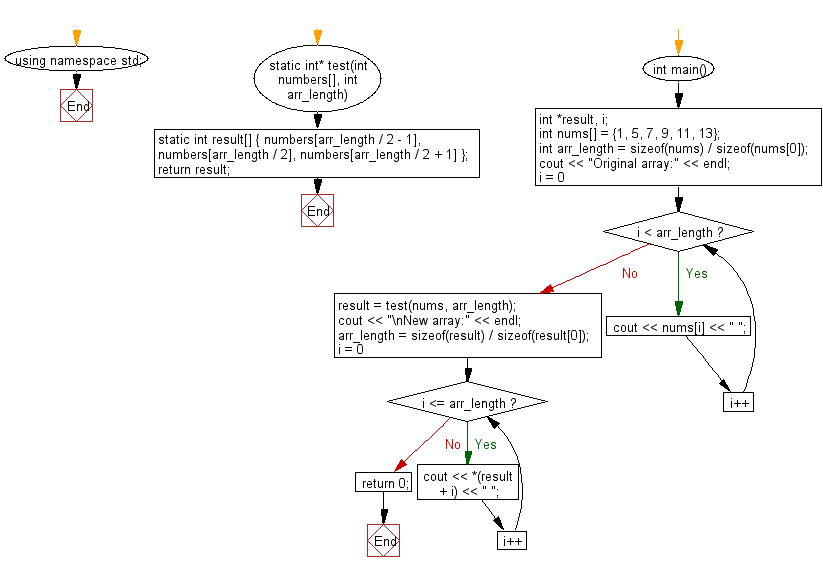
For more Practice: Solve these Related Problems:
- Write a C++ program to extract three consecutive middle elements from an array (length at least 3) and create a new array with them.
- Write a C++ program that reads an array and, if its length is odd and at least 3, outputs an array consisting of its middle three elements.
- Write a C++ program to determine the central three elements of an array and form a new array with these values.
- Write a C++ program that accepts an array of integers and creates a subarray of length 3 from its center, then prints the subarray.
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a C++ program to create a new array swapping the first and last elements of a given array of integers and length will be least 1.
Next: Write a C++ program to find the largest value from first, last, and middle elements of a given array of integers of odd length (atleast 1).
What is the difficulty level of this exercise?