C++ Exercises: Compute the sum of the two given arrays of integers, length 3 and find the array which has the largest sum
Sum Two Arrays and Find Larger
Write a C++ program to compute the sum of the two given arrays of integers, length 3 and find the array that has the largest sum.
Sample Solution:
C++ Code :
#include <iostream> // Including input-output stream header file
using namespace std; // Using standard namespace
static int *test(int nums1[], int nums2[]) // Function definition that compares sums of two arrays
{
return nums1[0] + nums1[1] + nums1[2] >= nums2[0] + nums2[1] + nums2[2] ? nums1 : nums2; // Returns pointer to the array with the larger sum
}
int main () {
int *p; // Declaring pointer variable p
int nums1[] = { 1, 5, 7 }; // Initializing array nums1 with values
int nums2[] = { 1, 5, 3 }; // Initializing array nums2 with values
int arr_length = sizeof(nums1) / sizeof(nums1[0]); // Calculating length of array nums1
p = test(nums1, nums2); // Calling the test function and storing the returned pointer in p
cout << "\nNew array: " << endl; // Printing a message
for ( int i = 0; i < arr_length; i++ ) { // Loop to print elements of the array pointed by p
cout << *(p + i) << " "; // Printing elements of the array pointed by p
}
return 0; // Returning 0 to indicate successful completion of the program
}
Sample Output:
New array: 1 5 7
Visual Presentation:
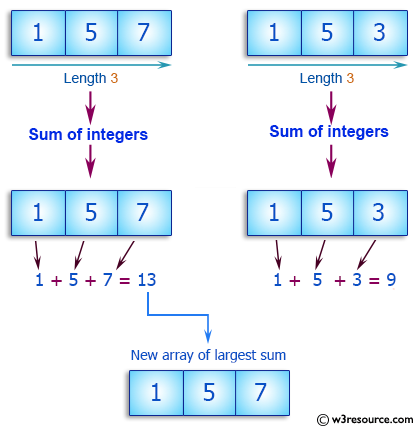
Flowchart:
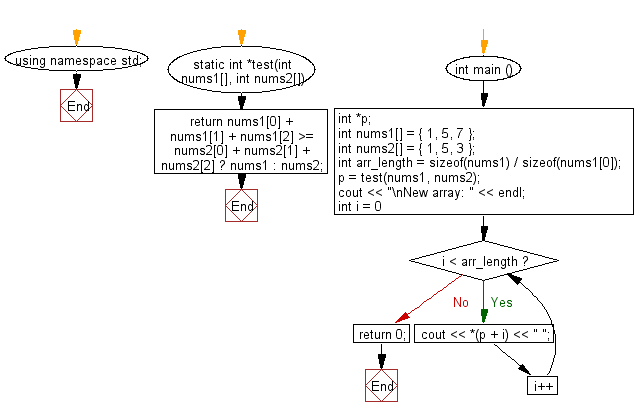
For more Practice: Solve these Related Problems:
- Write a C++ program to compute the sum of elements for two 3-element arrays and then output the array with the larger total sum.
- Write a C++ program that reads two arrays of length 3, calculates their sums, and prints the array corresponding to the higher sum.
- Write a C++ program to compare the sum of two fixed-size arrays and output the elements of the array that has the greater aggregate.
- Write a C++ program that computes sums for two 3-element arrays and uses a conditional operator to display the array with the larger sum.
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a C++ program to check a given array of integers, length 3 and create a new array. If there is a 5 in the given array immediately followed by a 7 then set 7 to 1.
Next: Write a C++ program to create an array taking two middle elements from a given array of integers of length even.
What is the difficulty level of this exercise?