C++ Exercises: Check a given array of integers and return true if the array contains 10 or 20 twice
Check Array for Two 10s or 20s
Write a C++ program to check a given array of integers and return true if the array contains 10 or 20 twice. The length of the array will be 0, 1, or 2.
Sample Solution:
C++ Code :
#include <iostream> // Including the input/output stream library
using namespace std; // Using the standard namespace
// Function to check if the array has exactly two elements and both are either 10 or both are 20
bool test(int nums[], int arr_length) {
return arr_length == 2
&& ((nums[0] == 10 && nums[1] == 10)
|| (nums[0] == 20 && nums[1] == 20));
}
// Main function
int main () {
int nums1[] = {12, 20}; // Define an array nums1
int arr_length = sizeof(nums1) / sizeof(nums1[0]); // Calculate the length of nums1
cout << test(nums1, arr_length) << endl; // Output the result of test function for nums1
int nums2[] = {20, 20}; // Define an array nums2
arr_length = sizeof(nums2) / sizeof(nums2[0]); // Calculate the length of nums2
cout << test(nums2, arr_length) << endl; // Output the result of test function for nums2
int nums3[] = {10, 10}; // Define an array nums3
cout << test(nums3, arr_length) << endl; // Output the result of test function for nums3
int nums4[] = {10}; // Define an array nums4
arr_length = sizeof(nums4) / sizeof(nums4[0]); // Calculate the length of nums4
cout << test(nums4, arr_length) << endl; // Output the result of test function for nums4
return 0; // Return statement indicating successful termination of the program
}
Sample Output:
0 1 1 0
Visual Presentation:
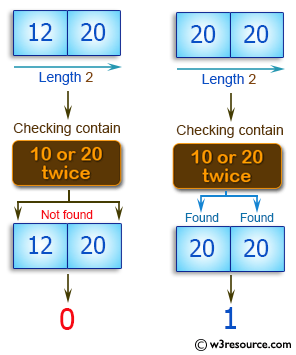
Flowchart:
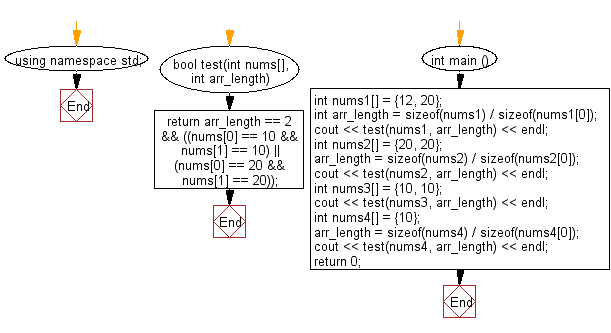
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a C++ program to check if a given array of integers and length 2, does not contain 15 or 20..
Next: Write a C++ program to check a given array of integers, length 3 and create a new array. If there is a 5 in the given array immediately followed by a 7 then set 7 to 1.
What is the difficulty level of this exercise?
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics