C++ Exercises: Create a new string with the last char added at the front and back of a given string of length 1 or more
C++ Basic Algorithm: Exercise-9 with Solution
Add Last Character to Front and Back
Write a C++ program to create a string with the last character added at the front and back of a given string of length 1 or more.
Sample Solution:
C++ Code :
#include <iostream>
using namespace std;
// Function to modify a string by appending its last character at both ends
string test(string str)
{
// Extract the last character of the string
string s = str.substr(str.length() - 1);
// Append the last character both at the beginning and end of the string
return s + str + s;
}
// Main function
int main()
{
cout << test("Red") << endl; // Output the result of test function with string "Red"
cout << test("Green") << endl; // Output the result of test function with string "Green"
cout << test("1") << endl; // Output the result of test function with string "1"
return 0; // Return 0 to indicate successful execution of the program
}
Sample Output:
dRedd nGreenn 111
Visual Presentation:
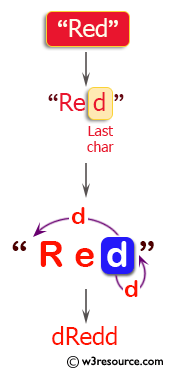
Flowchart:
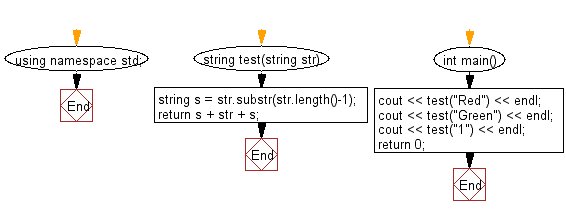
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a C++ program to create a new string which is 4 copies of the 2 front characters of a given string. If the given string length is less than 2 return the original string.
Next: Write a C++ program to check if a given positive number is a multiple of 3 or a multiple of 7.
What is the difficulty level of this exercise?
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://w3resource.com/cpp-exercises/basic-algorithm/cpp-basic-algorithm-exercise-9.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics