C++ Exercises: Compute the sum of the elements of a given array of integers
Sum of Array Elements
Write a C++ program to compute the sum of the elements of an array of integers.
Sample Solution:
C++ Code :
#include <iostream> // Including the input/output stream library
using namespace std; // Using the standard namespace
// Function that calculates the sum of elements in an integer array
int test(int a1[])
{
// Return the sum of the elements at indices 0 to 4 in array a1
return a1[0] + a1[1] + a1[2] + a1[3] + a1[4];
}
// Main function
int main()
{
int nums[] = {10, 20, 30, 40, 50}; // Define an array nums with 5 integer elements
cout << test(nums) << endl; // Output the result of test function for nums
int nums1[] = {10, 20, -30, -40, 50}; // Define an array nums1 with 5 integer elements (some negative)
cout << test(nums1) << endl; // Output the result of test function for nums1
return 0; // Return statement indicating successful termination of the program
}
Sample Output:
150 10
Visual Presentation:
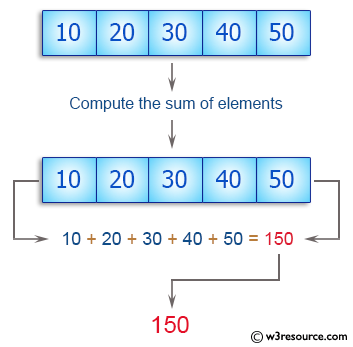
Flowchart:
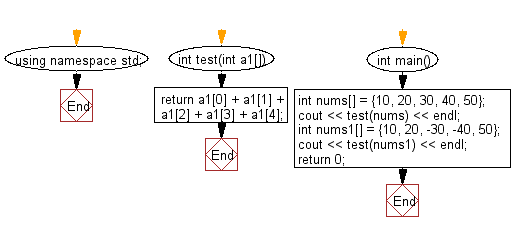
For more Practice: Solve these Related Problems:
- Write a C++ program to compute the sum of all elements in an integer array and print the result.
- Write a C++ program that reads an array of integers and outputs the total sum using a loop construct.
- Write a C++ program to calculate the aggregate of numbers in an array and display the result with a formatted message.
- Write a C++ program that sums the elements of an input array and prints the final sum, ensuring it handles negative numbers correctly.
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a C++ program to check two given arrays of integers of length 1 or more and return true if they have the same first element or they have the same last element.
Next: Write a C++ program to rotate the elements of a given array of integers (length 4 ) in left direction and return the new array.
What is the difficulty level of this exercise?