C++ Exercises: Create a new string using 3 copies of the first 2 characters of a given string
3 Copies of First 2 Characters or Full String
Write a C++ program to create a new string using 3 copies of the first 2 characters of a given string. If the length of the given string is less than 2 use the whole string.
Sample Solution:
C++ Code :
#include <iostream> // Including the input/output stream library
using namespace std; // Using the standard namespace
// Function that performs a specific operation on the input string based on its length
string test(string s1)
{
string extra_Front = ""; // Declare a string variable to store a substring
// Check if the length of s1 is less than 2
if (s1.length() < 2)
{
return s1 + s1 + s1; // Return concatenation of s1 with itself thrice if its length is less than 2
}
else
{
extra_Front = s1.substr(0, 2); // Store the substring of s1 from index 0 to 1 in extra_Front
return extra_Front + extra_Front + extra_Front; // Return concatenation of extra_Front with itself thrice
}
}
// Main function
int main()
{
cout << test("abc") << endl; // Output for "abc"
cout << test("Python") << endl; // Output for "Python"
cout << test("J") << endl; // Output for "J"
return 0; // Return statement indicating successful termination of the program
}
Sample Output:
ababab PyPyPy JJJ
Visual Presentation:
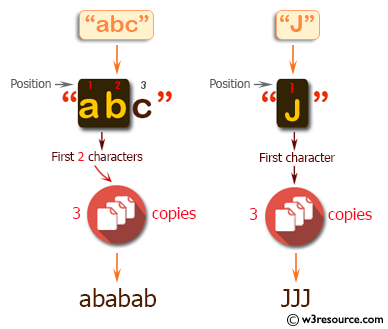
Flowchart:
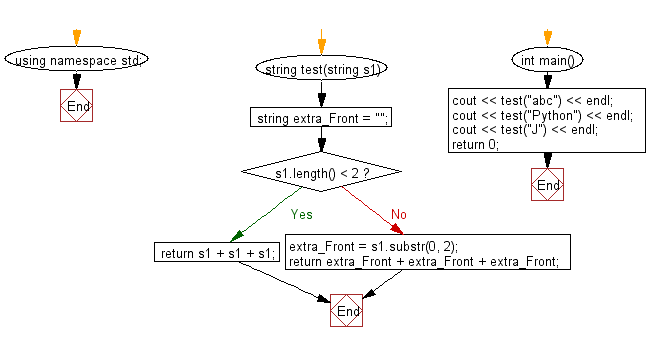
For more Practice: Solve these Related Problems:
- Write a C++ program that creates a new string consisting of three repetitions of the first two characters of an input string; if the string is shorter than 2 characters, repeat the entire string three times.
- Write a C++ program to read a string and output three copies of its first two letters, or the whole string repeated thrice if it is too short.
- Write a C++ program that constructs a new string by duplicating the first two characters three times; if the string length is less than 2, use the whole string for replication.
- Write a C++ program that checks the length of an input string and outputs a tripled copy of its first two characters, defaulting to the full string if necessary.
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a C++ program to concat two given strings. If the given strings have different length remove the characters from the longer string.
Next: Write a C++ program to create a new string from a given string. If the two characters of the given string from its beginning and end are same return the given string without the first two characters otherwise return the original string.
What is the difficulty level of this exercise?