C++ Exercises: Concat two given strings
Add Strings After Trimming Longer String
Write a C++ program to add two given strings. If the given strings have different lengths, remove the characters from the longer string.
Sample Solution:
C++ Code :
#include <iostream> // Including the input/output stream library
using namespace std; // Using the standard namespace
// Function that concatenates two strings based on their lengths
string test(string s1, string s2)
{
// Check if the length of s1 is less than the length of s2
if (s1.length() < s2.length())
{
// Concatenate s1 with a substring of s2 from (s2.length() - s1.length()) to the end of s2
return s1 + s2.substr(s2.length() - s1.length());
}
// Check if the length of s1 is greater than the length of s2
else if (s1.length() > s2.length())
{
// Concatenate a substring of s1 from (s1.length() - s2.length()) to the end of s1 with s2
return s1.substr(s1.length() - s2.length()) + s2;
}
else
{
// Concatenate s1 and s2 if their lengths are equal
return s1 + s2;
}
}
// Main function
int main()
{
cout << test("abc", "abcd") << endl; // Output for "abc" and "abcd"
cout << test("Python", "Python") << endl; // Output for "Python" and "Python"
cout << test("JS", "Python") << endl; // Output for "JS" and "Python"
return 0; // Return statement indicating successful termination of the program
}
Sample Output:
abcbcd PythonPython JSon
Visual Presentation:
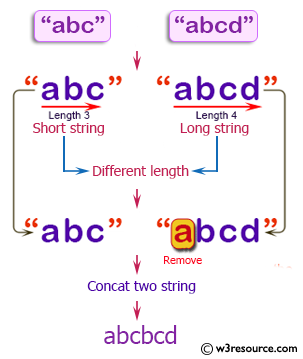
Flowchart:
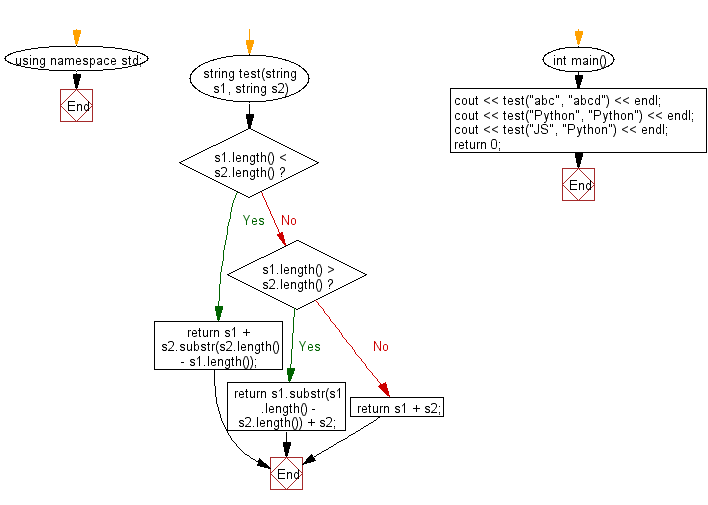
For more Practice: Solve these Related Problems:
- Write a C++ program that takes two strings and, if they are of unequal length, removes extra characters from the longer string before concatenating them.
- Write a C++ program to read two strings, trim the longer one to the length of the shorter, then concatenate the trimmed versions together.
- Write a C++ program that compares the lengths of two strings, trims the longer string to match the shorter one, and then outputs their concatenation.
- Write a C++ program to combine two strings after adjusting the longer string’s length to equal the shorter string’s length, then output the result.
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a C++ program to check whether the first two characters and last two characters of a given string are same.
Next: Write a C++ program to create a new string using 3 copies of the first 2 characters of a given string. If the length of the given string is less than 2 use the whole string.
What is the difficulty level of this exercise?