C++ Exercises: Create a new string taking the first character from a given string and the last character from another given string
First and Last Character from Two Strings
Write a C++ program to create a string taking the first character from a string and the last character from another given string. If the length of any given string is 0, use '#' as its missing character.
Sample Solution:
C++ Code :
#include <iostream> // Including the input/output stream library
using namespace std; // Using the standard namespace
// Function that constructs a string based on the first and last characters of two input strings
string test(string s1, string s2)
{
string lastChars = ""; // Initialize an empty string to store the constructed string
// Check if s1 has at least one character
if (s1.length() > 0)
{
lastChars += s1.substr(0, 1); // Append the first character of s1 to lastChars
}
else
{
lastChars += "#"; // If s1 is empty, append '#' to lastChars
}
// Check if s2 has at least one character
if (s2.length() > 0)
{
lastChars += s2.substr(s2.length() - 1); // Append the last character of s2 to lastChars
}
else
{
lastChars += "#"; // If s2 is empty, append '#' to lastChars
}
return lastChars; // Return the constructed string containing first character from s1 and last character from s2 (or '#' if any string is empty)
}
// Main function
int main()
{
cout << test("Hello", "Hi") << endl; // Output the result of test function with "Hello" and "Hi"
cout << test("Python", "PHP") << endl; // Output the result of test function with "Python" and "PHP"
cout << test("JS", "JS") << endl; // Output the result of test function with "JS" and "JS"
cout << test("Csharp", "") << endl; // Output the result of test function with "Csharp" and an empty string
return 0; // Return statement indicating successful termination of the program
}
Sample Output:
Hi PP JS C#
Visual Presentation:
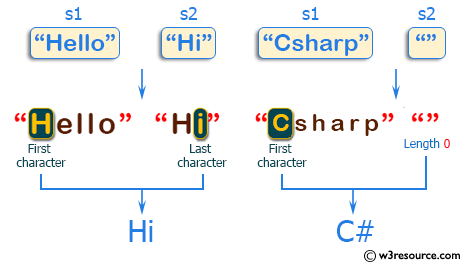
Flowchart:
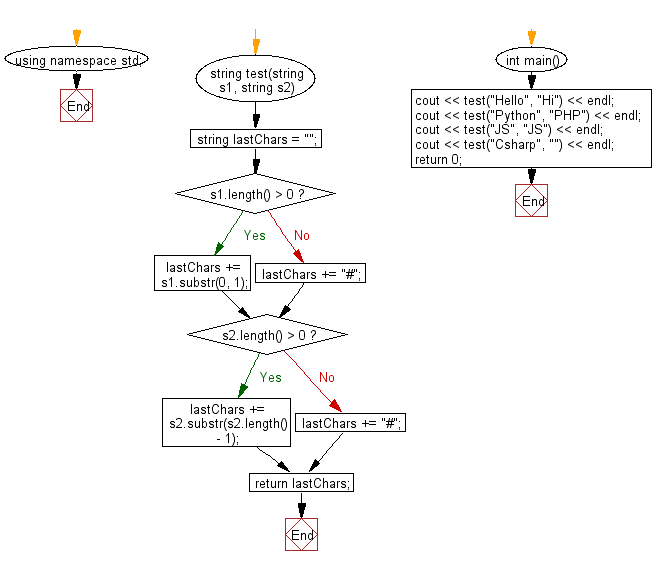
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a C++ program to create a new string of length 2, using first two characters of a given string. If the given string length is less than 2 use '#' as missing characters.
Next: Write a C++ program to create a new string from a given string after swapping last two characters.
What is the difficulty level of this exercise?
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics